Set Page Setup in Word Documents in C# and VB.NET
Page setup is a set of page layout and formatting options that affect how the content appears on the page. You can specify options like page orientation, size, and margins with page setup.
The GemBox.Document library allows you to control page setup in Word documents programmatically in C# and VB.NET. Each Section
has a PageSetup
property which defines display and layout settings of all pages that its content occupies.
The following example shows how to set some page setup properties like orientation, margins, and paper type.
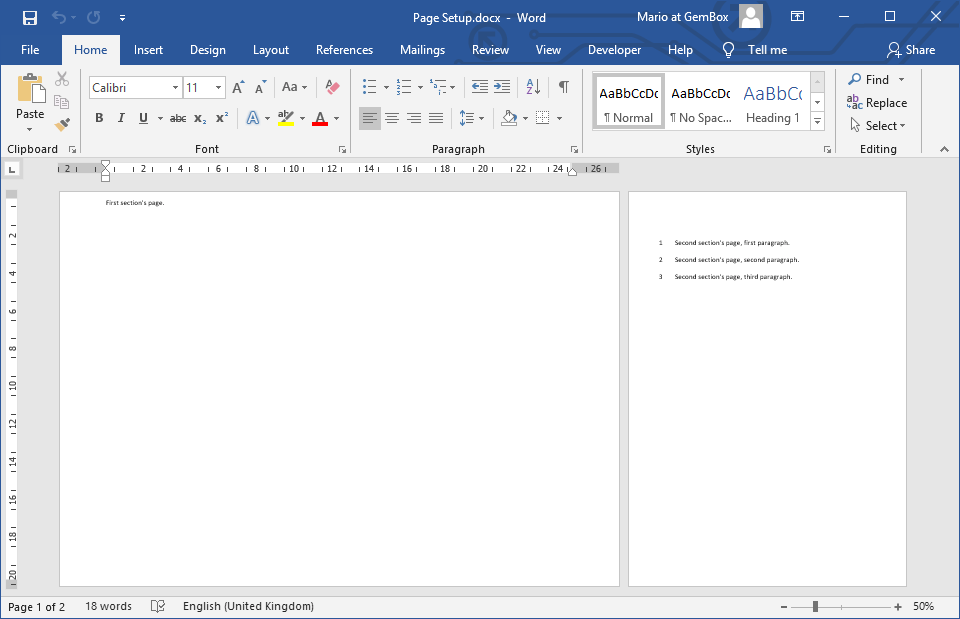
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
document.Sections.Add(
new Section(document,
new Paragraph(document, "First section's page.")));
document.Sections.Add(
new Section(document,
new Paragraph(document, "Second section's page, first paragraph."),
new Paragraph(document, "Second section's page, second paragraph."),
new Paragraph(document, "Second section's page, third paragraph.")));
var pageSetup1 = document.Sections[0].PageSetup;
var pageSetup2 = document.Sections[1].PageSetup;
// Set page orientation.
pageSetup1.Orientation = Orientation.Landscape;
// Set page margins.
pageSetup1.PageMargins.Top = 10;
pageSetup1.PageMargins.Bottom = 10;
// Set paper type.
pageSetup2.PaperType = PaperType.A5;
// Set line numbering.
pageSetup2.LineNumberRestartSetting = LineNumberRestartSetting.NewPage;
document.Save("Page Setup.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
document.Sections.Add(
New Section(document,
New Paragraph(document, "First section's page.")))
document.Sections.Add(
New Section(document,
New Paragraph(document, "Second section's page, first paragraph."),
New Paragraph(document, "Second section's page, second paragraph."),
New Paragraph(document, "Second section's page, third paragraph.")))
Dim pageSetup1 = document.Sections(0).PageSetup
Dim pageSetup2 = document.Sections(1).PageSetup
' Set page orientation.
pageSetup1.Orientation = Orientation.Landscape
' Set page margins.
pageSetup1.PageMargins.Top = 10
pageSetup1.PageMargins.Bottom = 10
' Set paper type.
pageSetup2.PaperType = PaperType.A5
' Set line numbering.
pageSetup2.LineNumberRestartSetting = LineNumberRestartSetting.NewPage
document.Save("Page Setup.%OutputFileType%")
End Sub
End Module