Merge Cells in Word Documents Using C# and VB.NET
With GemBox.Document you can merge TableCell
elements by specifying the number of rows and/or columns that the cell fills (the same way cells are merged in HTML).
The TableCell.RowSpan
property specifies the number of rows that a cell will occupy. If the value is greater than 1, the resulting merged cell stretches vertically.
The TableCell.ColumnSpan
property specifies the number of columns that a cell will occupy. The resulting merged cell stretches horizontally if the value is greater than 1.
The following example shows how you can create a table with merged cells that stretch across multiple rows, multiple columns, or both.
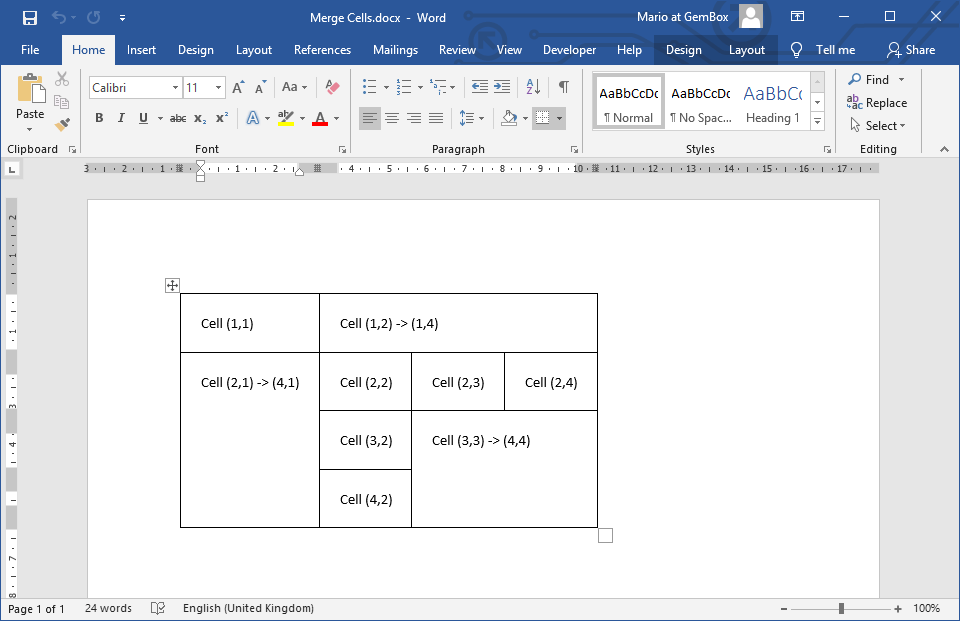
using GemBox.Document;
using GemBox.Document.Tables;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var table = new Table(document,
new TableRow(document,
new TableCell(document, new Paragraph(document, "Cell (1,1)")),
new TableCell(document, new Paragraph(document, "Cell (1,2) -> (1,4)"))
{
ColumnSpan = 3
}),
new TableRow(document,
new TableCell(document, new Paragraph(document, "Cell (2,1) -> (4,1)"))
{
RowSpan = 3
},
new TableCell(document, new Paragraph(document, "Cell (2,2)")),
new TableCell(document, new Paragraph(document, "Cell (2,3)")),
new TableCell(document, new Paragraph(document, "Cell (2,4)"))),
new TableRow(document,
new TableCell(document, new Paragraph(document, "Cell (3,2)")),
new TableCell(document, new Paragraph(document, "Cell (3,3) -> (4,4)"))
{
ColumnSpan = 2,
RowSpan = 2
}),
new TableRow(document,
new TableCell(document, new Paragraph(document, "Cell (4,2)"))));
table.TableFormat.DefaultCellPadding = new Padding(15);
document.Sections.Add(new Section(document, table));
document.Save("Merge Cells.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Tables
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim table As New Table(document,
New TableRow(document,
New TableCell(document, New Paragraph(document, "Cell (1,1)")),
New TableCell(document, New Paragraph(document, "Cell (1,2) -> (1,4)")) With
{
.ColumnSpan = 3
}),
New TableRow(document,
New TableCell(document, New Paragraph(document, "Cell (2,1) -> (4,1)")) With
{
.RowSpan = 3
},
New TableCell(document, New Paragraph(document, "Cell (2,2)")),
New TableCell(document, New Paragraph(document, "Cell (2,3)")),
New TableCell(document, New Paragraph(document, "Cell (2,4)"))),
New TableRow(document,
New TableCell(document, New Paragraph(document, "Cell (3,2)")),
New TableCell(document, New Paragraph(document, "Cell (3,3) -> (4,4)")) With
{
.ColumnSpan = 2,
.RowSpan = 2
}),
New TableRow(document,
New TableCell(document, New Paragraph(document, "Cell (4,2)"))))
table.TableFormat.DefaultCellPadding = New Padding(15)
document.Sections.Add(New Section(document, table))
document.Save("Merge Cells.%OutputFileType%")
End Sub
End Module
In Word files you can Merge table cells into one cell. The resulting merged cell will stretch across all the selected cells and contain their combined content.
To achieve this programmatically, you need to retrieve the cells you want to merge from the Table
element. The first cell, the one in the upper left corner of the targeted cells, will be the resulting merged cell, so you need to specify the desired row and column-span on it.
You need to remove the rest of the cells from their TableCell.ParentCollection
. Additionally, you can copy their TableCell.Blocks
content and add it to the merged cell's content.