Headers and Footers
Headers and footers are parts of Word documents usually used for complementary information such as the date, page number, author's information, or company logos. You can place a header's content at the top of the page and add a footer's content at the bottom.
With GemBox.Document, you can add footers and headers programmatically to your Word documents in C# and VB.NET.
When using the GemBox.Document you can represent headers and footers with HeaderFooter
elements, repeated on each page. They are defined for each Section
element, whose content can occupy one or more pages. Each Section.HeadersFooters
collection can contain only one instance of each HeaderFooterType
type.
In other words, you can define different headers and footers for the first page, even pages, and odd pages. If you need different headers and footers on various pages, you can set up multiple sections and create the desired headers and footers for each one of them.
The following example shows how you can add all types of headers and footers to a document section.
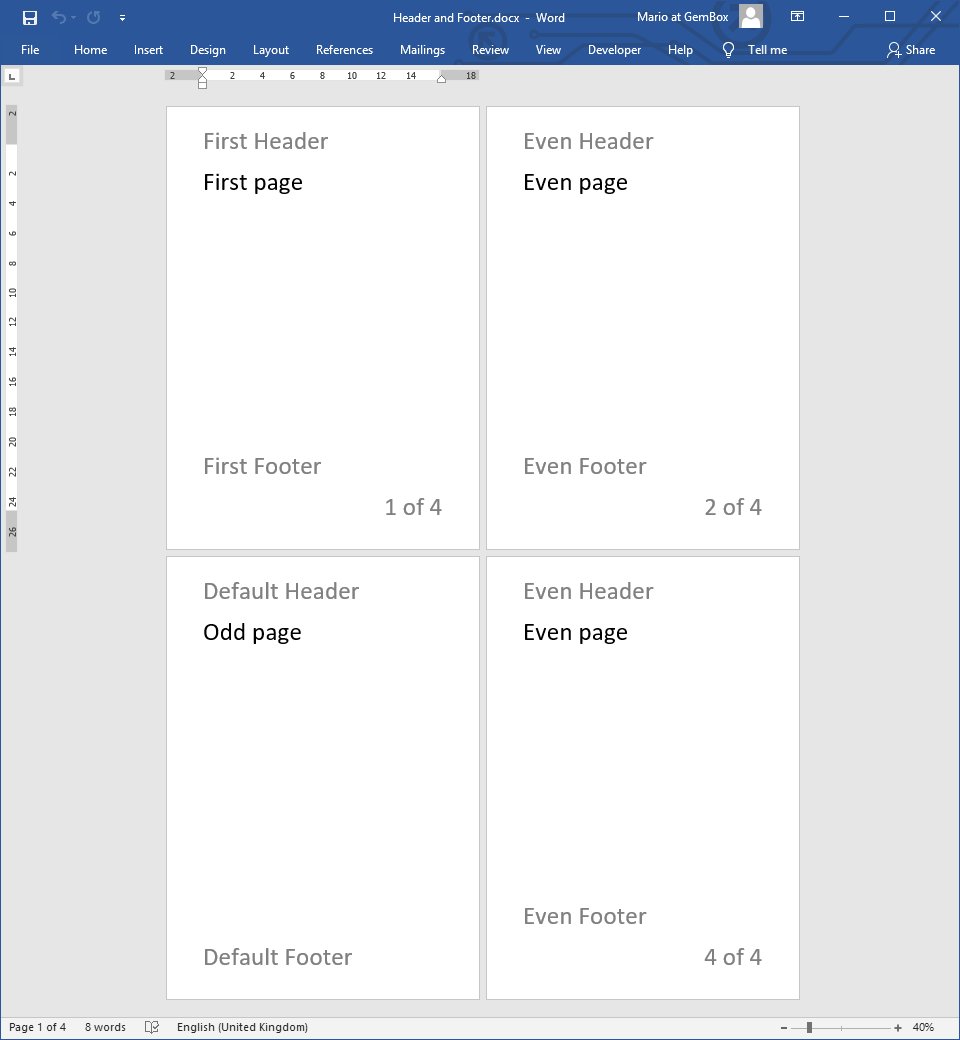
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
document.DefaultCharacterFormat.Size = 48;
var section = new Section(document,
new Paragraph(document, "First page"),
new Paragraph(document, new SpecialCharacter(document, SpecialCharacterType.PageBreak)),
new Paragraph(document, "Even page"),
new Paragraph(document, new SpecialCharacter(document, SpecialCharacterType.PageBreak)),
new Paragraph(document, "Odd page"),
new Paragraph(document, new SpecialCharacter(document, SpecialCharacterType.PageBreak)),
new Paragraph(document, "Even page"));
document.Sections.Add(section);
// Add default (odd) header.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.HeaderDefault,
new Paragraph(document, "Default Header")));
// Add default (odd) footer with page number.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.FooterDefault,
new Paragraph(document, "Default Footer")));
// Add first header.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.HeaderFirst,
new Paragraph(document, "First Header")));
// Add first footer.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.FooterFirst,
new Paragraph(document, "First Footer"),
new Paragraph(document,
new Field(document, FieldType.Page),
new Run(document, " of "),
new Field(document, FieldType.NumPages))
{
ParagraphFormat = new ParagraphFormat() { Alignment = HorizontalAlignment.Right }
}));
// Add even header.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.HeaderEven,
new Paragraph(document, "Even Header")));
// Add even footer with page number.
section.HeadersFooters.Add(
new HeaderFooter(document, HeaderFooterType.FooterEven,
new Paragraph(document, "Even Footer"),
new Paragraph(document,
new Field(document, FieldType.Page),
new Run(document, " of "),
new Field(document, FieldType.NumPages))
{
ParagraphFormat = new ParagraphFormat() { Alignment = HorizontalAlignment.Right }
}));
document.Save("Header and Footer.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
document.DefaultCharacterFormat.Size = 48
Dim section As New Section(document,
New Paragraph(document, "First page"),
New Paragraph(document, New SpecialCharacter(document, SpecialCharacterType.PageBreak)),
New Paragraph(document, "Even page"),
New Paragraph(document, New SpecialCharacter(document, SpecialCharacterType.PageBreak)),
New Paragraph(document, "Odd page"),
New Paragraph(document, New SpecialCharacter(document, SpecialCharacterType.PageBreak)),
New Paragraph(document, "Even page"))
document.Sections.Add(section)
' Add default (odd) header.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.HeaderDefault,
New Paragraph(document, "Default Header")))
' Add default (odd) footer with page number.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.FooterDefault,
New Paragraph(document, "Default Footer")))
' Add first header.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.HeaderFirst,
New Paragraph(document, "First Header")))
' Add first footer.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.FooterFirst,
New Paragraph(document, "First Footer"),
New Paragraph(document,
New Field(document, FieldType.Page),
New Run(document, " of "),
New Field(document, FieldType.NumPages)) With
{
.ParagraphFormat = New ParagraphFormat() With {.Alignment = HorizontalAlignment.Right}
}))
' Add even header.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.HeaderEven,
New Paragraph(document, "Even Header")))
' Add even footer with page number.
section.HeadersFooters.Add(
New HeaderFooter(document, HeaderFooterType.FooterEven,
New Paragraph(document, "Even Footer"),
New Paragraph(document,
New Field(document, FieldType.Page),
New Run(document, " of "),
New Field(document, FieldType.NumPages)) With
{
.ParagraphFormat = New ParagraphFormat() With {.Alignment = HorizontalAlignment.Right}
}))
document.Save("Header and Footer.%OutputFileType%")
End Sub
End Module