Word Editor in Windows Forms
Rich editing controls for desktop applications often use the RTF format as their internal object model or provide support for importing and exporting RTF content.
GemBox.Document supports both reading and writing RTF files, which enables you to combine it with such controls to create an advanced Word editor, one that's capable of saving to PDF, printing, mail merging, and a lot more.
The following example shows interoperability between GemBox.Document library and Windows Forms RichTextBox
control via RTF. The example also implements some common Word functionalities for text editing like modifying, styling, copying, and pasting.
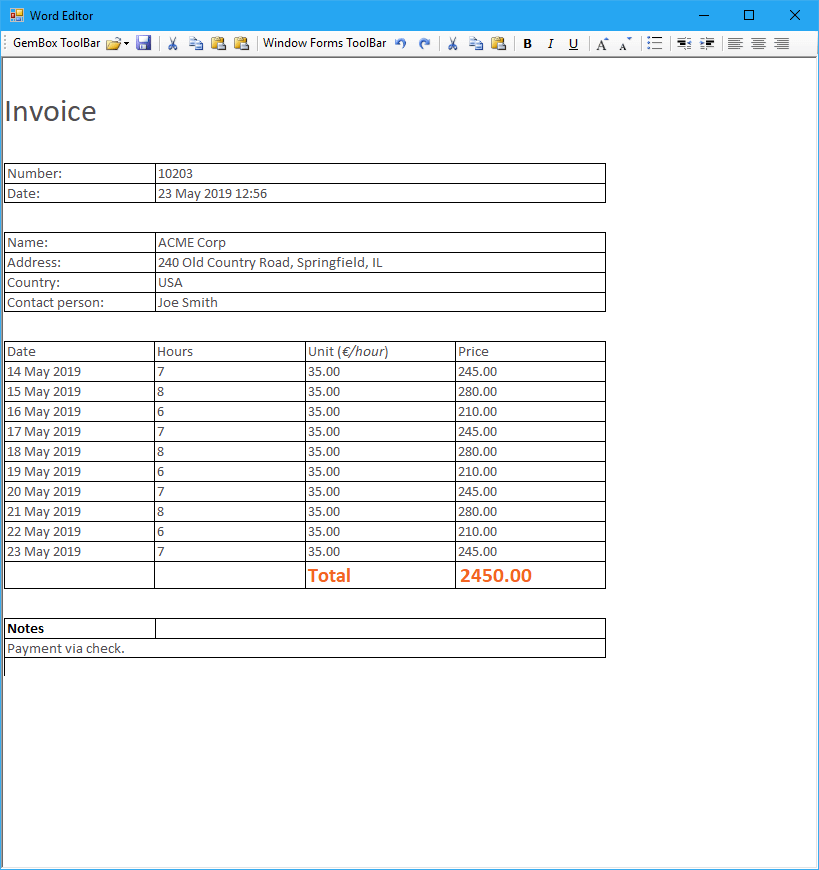
using GemBox.Document;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
namespace WindowsFormsRichTextEditor
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
private void menuItemOpen_Click(object sender, EventArgs e)
{
var dialog = new OpenFileDialog()
{
AddExtension = true,
Filter =
"All Documents (*.docx;*.docm;*.doc;*.dotx;*.dotm;*.dot;*.htm;*.html;*.rtf;*.xml;*.txt)|*.docx;*.docm;*.dotx;*.dotm;*.doc;*.dot;*.htm;*.html;*.rtf;*.xml;*.txt|" +
"Word Documents (*.docx)|*.docx|" +
"Word Macro-Enabled Documents (*.docm)|*.docm|" +
"Word 97-2003 Documents (*.doc)|*.doc|" +
"Word Templates (*.dotx)|*.dotx|" +
"Word Macro-Enabled Templates (*.dotm)|*.dotm|" +
"Word 97-2003 Templates (*.dot)|*.dot|" +
"Web Pages (*.htm;*.html)|*.htm;*.html|" +
"Rich Text Format (*.rtf)|*.rtf|" +
"Flat OPC (*.xml)|*.xml|" +
"Plain Text (*.txt)|*.txt"
};
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
using (var stream = new MemoryStream())
{
// Convert input file to RTF stream.
DocumentModel.Load(dialog.FileName).Save(stream, SaveOptions.RtfDefault);
stream.Position = 0;
// Load RTF stream into RichTextBox.
this.richTextBox.LoadFile(stream, RichTextBoxStreamType.RichText);
}
}
private void btnSave_Click(object sender, EventArgs e)
{
var dialog = new SaveFileDialog()
{
AddExtension = true,
Filter =
"Word Document (*.docx)|*.docx|" +
"Word Macro-Enabled Document (*.docm)|*.docm|" +
"Word Template (*.dotx)|*.dotx|" +
"Word Macro-Enabled Template (*.dotm)|*.dotm|" +
"PDF (*.pdf)|*.pdf|" +
"XPS Document (*.xps)|*.xps|" +
"Web Page (*.htm;*.html)|*.htm;*.html|" +
"Single File Web Page (*.mht;*.mhtml)|*.mht;*.mhtml|" +
"Rich Text Format (*.rtf)|*.rtf|" +
"Flat OPC (*.xml)|*.xml|" +
"Plain Text (*.txt)|*.txt|" +
"Image (*.png;*.jpg;*.jpeg;*.gif;*.bmp;*.tif;*.tiff;*.wdp,*.svg)|*.png;*.jpg;*.jpeg;*.gif;*.bmp;*.tif;*.tiff;*.wdp;*.svg"
};
if (dialog.ShowDialog(this) == System.Windows.Forms.DialogResult.OK)
using (var stream = new MemoryStream())
{
// Save RichTextBox content to RTF stream.
this.richTextBox.SaveFile(stream, RichTextBoxStreamType.RichText);
stream.Position = 0;
// Convert RTF stream to output format.
DocumentModel.Load(stream, LoadOptions.RtfDefault).Save(dialog.FileName);
Process.Start(dialog.FileName);
}
}
private void DoGemBoxCopy()
{
using (var stream = new MemoryStream())
{
// Save RichTextBox selection to RTF stream.
var writer = new StreamWriter(stream);
writer.Write(this.richTextBox.SelectedRtf);
writer.Flush();
stream.Position = 0;
// Save RTF stream to clipboard.
DocumentModel.Load(stream, LoadOptions.RtfDefault).Content.SaveToClipboard();
}
}
private void DoGemBoxPaste(bool prepend)
{
using (var stream = new MemoryStream())
{
// Save RichTextBox content to RTF stream.
var writer = new StreamWriter(stream);
writer.Write(this.richTextBox.SelectedRtf);
writer.Flush();
stream.Position = 0;
// Load document from RTF stream and prepend or append clipboard content to it.
var document = DocumentModel.Load(stream, LoadOptions.RtfDefault);
var content = document.Content;
(prepend ? content.Start : content.End).LoadFromClipboard();
stream.Position = 0;
// Save document to RTF stream.
document.Save(stream, SaveOptions.RtfDefault);
stream.Position = 0;
// Load RTF stream into RichTextBox.
var reader = new StreamReader(stream);
this.richTextBox.SelectedRtf = reader.ReadToEnd();
}
}
}
}
Imports GemBox.Document
Imports System
Imports System.Collections.Generic
Imports System.Diagnostics
Imports System.Drawing
Imports System.IO
Imports System.Windows.Forms
Partial Public Class MainForm
Inherits Form
Public Sub New()
InitializeComponent()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
End Sub
Private Sub menuItemOpen_Click(sender As Object, e As EventArgs)
Dim dialog = New OpenFileDialog() With {
.AddExtension = True,
.Filter =
"All Documents (*.docx;*.docm;*.doc;*.dotx;*.dotm;*.dot;*.htm;*.html;*.rtf;*.xml;*.txt)|*.docx;*.docm;*.dotx;*.dotm;*.doc;*.dot;*.htm;*.html;*.rtf;*.xml;*.txt|" &
"Word Documents (*.docx)|*.docx|" &
"Word Macro-Enabled Documents (*.docm)|*.docm|" &
"Word 97-2003 Documents (*.doc)|*.doc|" &
"Word Templates (*.dotx)|*.dotx|" &
"Word Macro-Enabled Templates (*.dotm)|*.dotm|" &
"Word 97-2003 Templates (*.dot)|*.dot|" &
"Web Pages (*.htm;*.html)|*.htm;*.html|" &
"Rich Text Format (*.rtf)|*.rtf|" &
"Flat OPC (*.xml)|*.xml|" &
"Plain Text (*.txt)|*.txt"
}
If dialog.ShowDialog() = System.Windows.Forms.DialogResult.OK Then
Using stream = New MemoryStream()
' Convert input file to RTF stream.
DocumentModel.Load(dialog.FileName).Save(stream, SaveOptions.RtfDefault)
stream.Position = 0
' Load RTF stream into RichTextBox.
Me.richTextBox.LoadFile(stream, RichTextBoxStreamType.RichText)
End Using
End If
End Sub
Private Sub btnSave_Click(sender As Object, e As EventArgs)
Dim dialog = New SaveFileDialog() With {
.AddExtension = True,
.Filter =
"Word Document (*.docx)|*.docx|" &
"Word Macro-Enabled Document (*.docm)|*.docm|" &
"Word Template (*.dotx)|*.dotx|" +
"Word Macro-Enabled Template (*.dotm)|*.dotm|" &
"PDF (*.pdf)|*.pdf|" &
"XPS Document (*.xps)|*.xps|" &
"Web Page (*.htm;*.html)|*.htm;*.html|" &
"Single File Web Page (*.mht;*.mhtml)|*.mht;*.mhtml|" &
"Rich Text Format (*.rtf)|*.rtf|" &
"Flat OPC (*.xml)|*.xml|" &
"Plain Text (*.txt)|*.txt|" &
"Image (*.png;*.jpg;*.jpeg;*.gif;*.bmp;*.tif;*.tiff;*.wdp,*.svg)|*.png;*.jpg;*.jpeg;*.gif;*.bmp;*.tif;*.tiff;*.wdp;*.svg"
}
If dialog.ShowDialog(Me) = System.Windows.Forms.DialogResult.OK Then
Using stream = New MemoryStream()
' Save RichTextBox content to RTF stream.
Me.richTextBox.SaveFile(stream, RichTextBoxStreamType.RichText)
stream.Position = 0
' Convert RTF stream to output format.
DocumentModel.Load(stream, LoadOptions.RtfDefault).Save(dialog.FileName)
Process.Start(dialog.FileName)
End Using
End If
End Sub
Private Sub DoGemBoxCopy()
Using stream = New MemoryStream()
' Save RichTextBox selection to RTF stream.
Dim writer = New StreamWriter(stream)
writer.Write(Me.richTextBox.SelectedRtf)
writer.Flush()
stream.Position = 0
' Save RTF stream to clipboard.
DocumentModel.Load(stream, LoadOptions.RtfDefault).Content.SaveToClipboard()
End Using
End Sub
Private Sub DoGemBoxPaste(prepend As Boolean)
Using stream = New MemoryStream()
' Save RichTextBox content to RTF stream.
Dim writer = New StreamWriter(stream)
writer.Write(Me.richTextBox.SelectedRtf)
writer.Flush()
stream.Position = 0
' Load document from RTF stream and prepend or append clipboard content to it.
Dim document = DocumentModel.Load(stream, LoadOptions.RtfDefault)
Dim content = document.Content
Dim contentPosition As ContentPosition = If(prepend, content.Start, content.End)
contentPosition.LoadFromClipboard()
stream.Position = 0
' Save document to RTF stream.
document.Save(stream, SaveOptions.RtfDefault)
stream.Position = 0
' Load RTF stream into RichTextBox.
Dim reader = New StreamReader(stream)
Me.richTextBox.SelectedRtf = reader.ReadToEnd()
End Using
End Sub
End Class