Conditional fields during mail merge in C# and VB.NET
GemBox.Document supports conditional mail merging with conditional fields (IF fields) in Word documents. You can handle this action programmatically in C# and VB.NET.
When using IF fields, you can compare two text values in the mail merge process and merge or insert the appropriate result text.
The following example shows how to perform a conditional mail merge and get resolved IF field results.
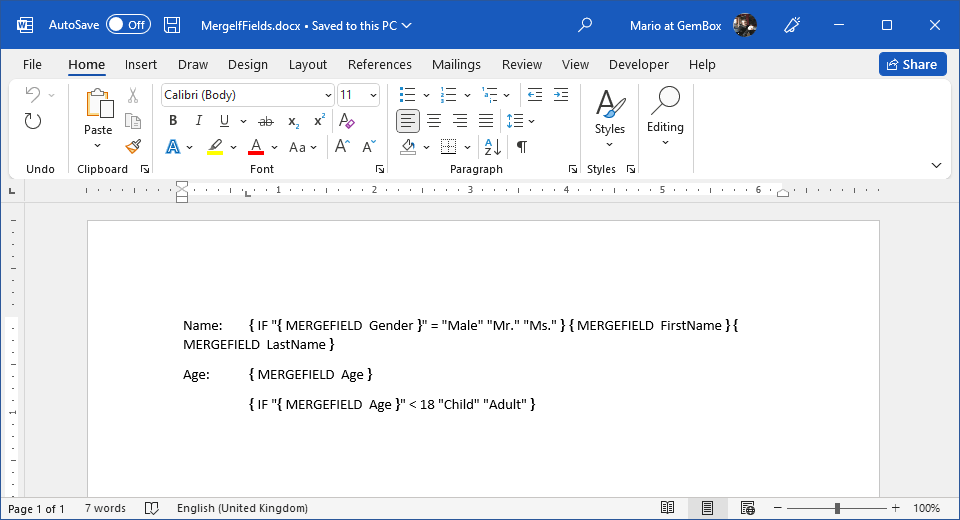
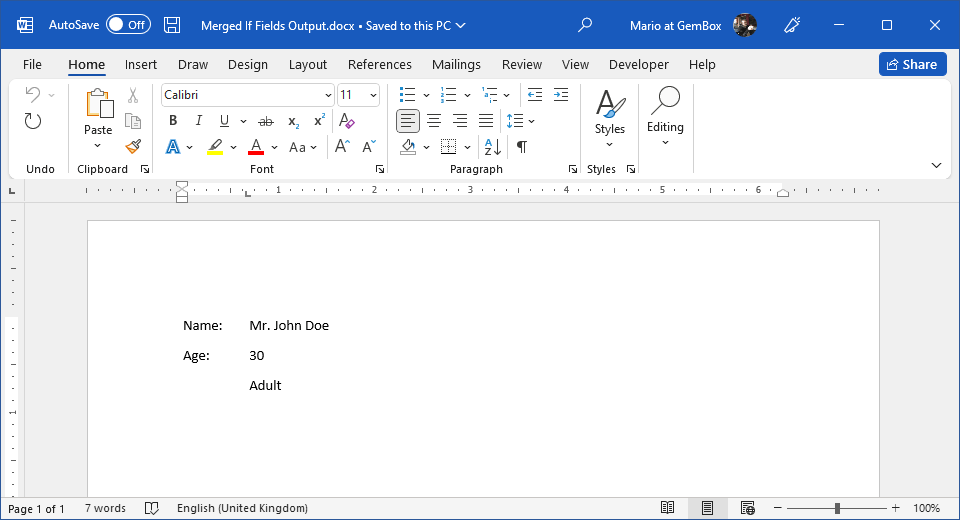
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
var data = new
{
FirstName = "John",
LastName = "Doe",
Gender = "Male",
Age = 30
};
document.MailMerge.Execute(data);
document.Save("Merged If Fields Output.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%InputFileName%")
Dim data = New With
{
.FirstName = "John",
.LastName = "Doe",
.Gender = "Male",
.Age = 30
}
document.MailMerge.Execute(data)
document.Save("Merged If Fields Output.%OutputFileType%")
End Sub
End Module
The IF field's code uses the following syntax:
{ IF "Value1" Operator "Value2" "TrueText" "FalseText" }
Each part, except Operator
, can contain text and other Field
elements. After executing a mail merge process, the result is either TrueText
or FalseText
, based on the used comparison operator.
Operator | Description |
---|---|
= | Equal |
<> | Not equal |
< | Less than |
> | Greater than |
<= | Less than or equal |
>= | Greater than or equal |
You can also combine COMPARE
and FORMULA
fields and use them inside one another to create more complex scenarios:
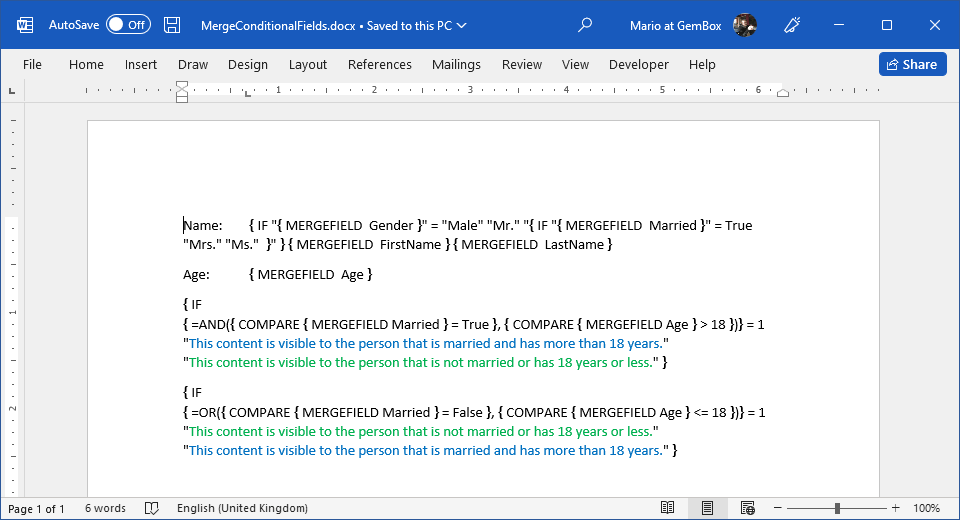
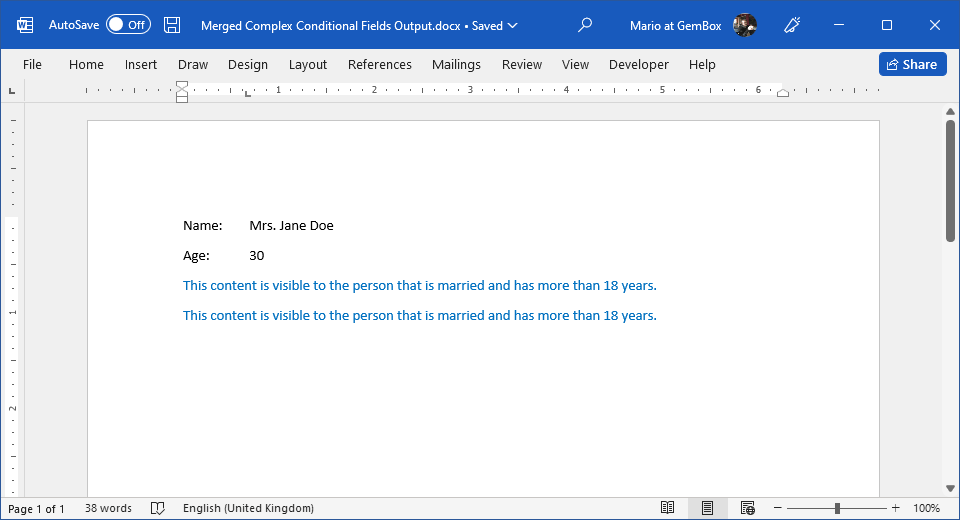
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
var data = new
{
FirstName = "Jane",
LastName = "Doe",
Gender = "Female",
Age = 30,
Married = true
};
document.MailMerge.Execute(data);
document.Save("Merged Complex Conditional Fields Output.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%InputFileName%")
Dim data = New With
{
.FirstName = "Jane",
.LastName = "Doe",
.Gender = "Female",
.Age = 30,
.Married = True
}
document.MailMerge.Execute(data)
document.Save("Merged Complex Conditional Fields Output.%OutputFileType%")
End Sub
End Module