Get Content in Word Files Using C# and VB.NET
GemBox.Document allows you to get content in Word files programmatically in your C# and VB.NET applications.
The content of a document is represented with the ContentRange
class which is exposed to the following members:
- The
Element.Content
property so that every document's element has it. - The
ElementCollection.Content
property so that every document's collection has it. - The
Bookmark.GetContent
method so that bookmarked content can be retrieved, modified or deleted.
The following example shows how you can retrieve the plain text representation of document elements by using the ContentRange.ToString
method.
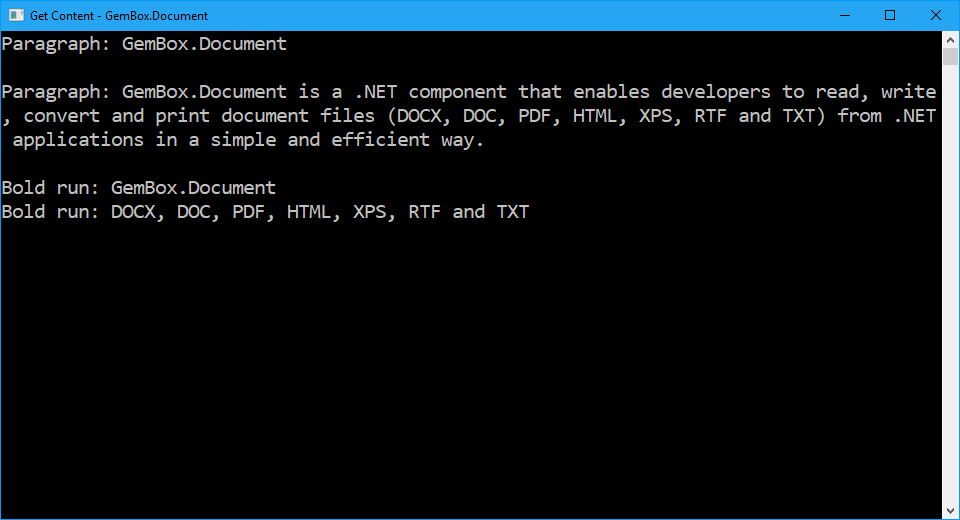
using System;
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
// Get content from each paragraph.
foreach (Paragraph paragraph in document.GetChildElements(true, ElementType.Paragraph))
Console.WriteLine($"Paragraph: {paragraph.Content.ToString()}");
// Get content from each bold run.
foreach (Run run in document.GetChildElements(true, ElementType.Run))
if (run.CharacterFormat.Bold)
Console.WriteLine($"Bold run: {run.Content.ToString()}");
}
}
Imports System
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
' Get content from each paragraph.
For Each paragraph As Paragraph In document.GetChildElements(True, ElementType.Paragraph)
Console.WriteLine($"Paragraph: {paragraph.Content.ToString()}")
Next
' Get content from each bold run.
For Each run As Run In document.GetChildElements(True, ElementType.Run)
If run.CharacterFormat.Bold Then
Console.WriteLine($"Bold run: {run.Content.ToString()}")
End If
Next
End Sub
End Module