Generate Barcodes and QR Codes
The following example shows how you can use GemBox.Document to insert a QR Code into a document using a Field element and save the document to PDF or other file formats in C# and VB.NET.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var qrCodeValue = "%BarcodeValue%";
var qrCodeField = new Field(document, FieldType.DisplayBarcode, $"{qrCodeValue} QR");
document.Sections.Add(
new Section(document,
new Paragraph(document, qrCodeField)));
document.Save("QR Code Output.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim qrCodeValue = "%BarcodeValue%"
Dim qrCodeField As New Field(document, FieldType.DisplayBarcode, $"{qrCodeValue} QR")
document.Sections.Add(
New Section(document,
New Paragraph(document, qrCodeField)))
document.Save("QR Code Output.%OutputFileType%")
End Sub
End Module
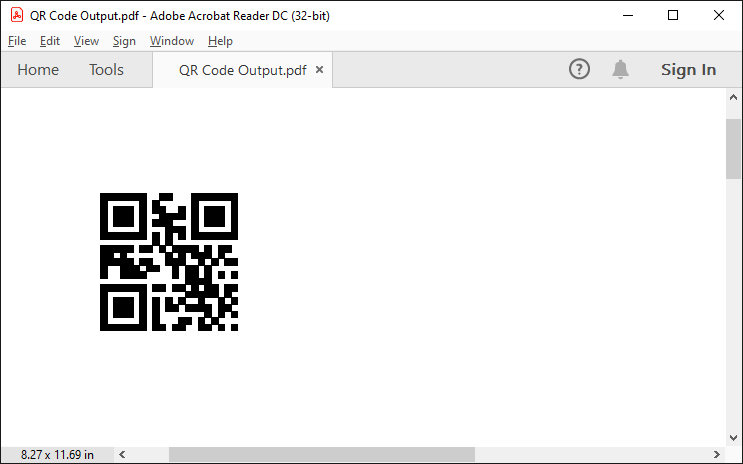
Currently, GemBox.Document supports the following barcode types:
- QR Code
- Code 39 (also called Code 3 of 9)
- Code 128
- EAN 13 / JAN 13
- EAN 8 / JAN 8
- UPCA
- ITF14
- NW7 (Codabar)
Customize barcodes
The following example shows how you can use a simple utility method to create various barcodes with advanced options:
using GemBox.Document;
using System.Text;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var ean13 = CreateBarcodeField(
document,
barcodeType: "EAN13",
barcodeValue: "5901234123457",
heightInPoints: 100,
showLabel: true);
var upca = CreateBarcodeField(
document,
barcodeType: "UPCA",
barcodeValue: "123456789104",
showLabel: true);
var code128 = CreateBarcodeField(
document,
barcodeType: "Code128",
barcodeValue: "012345678",
foregroundColor: "0xff7225",
backgroundColor: "0x25b2ff");
document.Sections.Add(
new Section(document,
new Paragraph(document, "EAN13 Code:"),
new Paragraph(document, ean13),
new Paragraph(document, "UPCA Code:"),
new Paragraph(document, upca),
new Paragraph(document, "Code 128:"),
new Paragraph(document, code128)));
document.Save("Barcodes.%OutputFileType%");
}
static Field CreateBarcodeField(DocumentModel document, string barcodeType, string barcodeValue,
int? heightInPoints = null, string foregroundColor = null,
string backgroundColor = null, bool showLabel = false)
{
var instructionText = new StringBuilder();
instructionText.Append(barcodeValue).Append(' ').Append(barcodeType);
if (heightInPoints.HasValue)
instructionText.Append(" \\h ").Append(LengthUnitConverter.Convert(heightInPoints.Value, LengthUnit.Point, LengthUnit.Twip));
if (foregroundColor != null)
instructionText.Append(" \\f ").Append(foregroundColor);
if (backgroundColor != null)
instructionText.Append(" \\b ").Append(backgroundColor);
if (showLabel)
instructionText.Append(" \\t");
return new Field(document, FieldType.DisplayBarcode, instructionText.ToString());
}
}
Imports GemBox.Document
Imports System.Text
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim ean13 = CreateBarcodeField(
document,
barcodeType:="EAN13",
barcodeValue:="5901234123457",
heightInPoints:=100,
showLabel:=True)
Dim upca = CreateBarcodeField(
document,
barcodeType:="UPCA",
barcodeValue:="123456789104",
showLabel:=True)
Dim code128 = CreateBarcodeField(
document,
barcodeType:="Code128",
barcodeValue:="012345678",
foregroundColor:="0xff7225",
backgroundColor:="0x25b2ff")
document.Sections.Add(
New Section(document,
New Paragraph(document, "EAN13:"),
New Paragraph(document, ean13),
New Paragraph(document, "UPCA:"),
New Paragraph(document, upca),
New Paragraph(document, "Code 128:"),
New Paragraph(document, code128)))
document.Save("Barcodes.%OutputFileType%")
End Sub
Function CreateBarcodeField(document As DocumentModel, barcodeType As String, barcodeValue As String,
Optional heightInPoints As Integer? = Nothing, Optional foregroundColor As String = Nothing,
Optional backgroundColor As String = Nothing, Optional showLabel As Boolean = False) As Field
Dim instructionText As New StringBuilder()
instructionText.Append(barcodeValue).Append(" "c).Append(barcodeType)
If heightInPoints.HasValue Then instructionText.Append(" \h ").Append(LengthUnitConverter.Convert(heightInPoints.Value, LengthUnit.Point, LengthUnit.Twip))
If foregroundColor IsNot Nothing Then instructionText.Append(" \f ").Append(foregroundColor)
If backgroundColor IsNot Nothing Then instructionText.Append(" \b ").Append(backgroundColor)
If showLabel Then instructionText.Append(" \t")
Return New Field(document, FieldType.DisplayBarcode, instructionText.ToString())
End Function
End Module
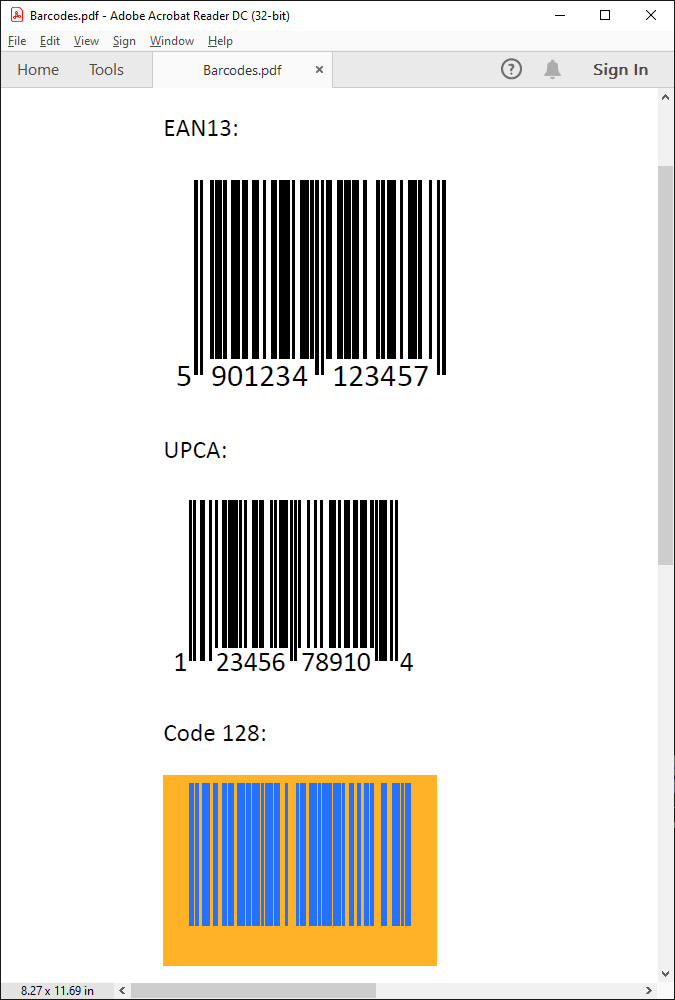
You can customize the resulting barcode with the following field switches:
- \h - the height of the barcode in twips.
- \s - the scaling factor of the QR Code.
- \q - the error correction level of the QR Code.
- \f - the foreground color of the barcode. MS Word interprets colors in the BGR format, so the least significant bytes represent the red color component and the most significant bytes represent the blue color component. For example, 0x0000FF represents the red color instead of blue.
- \b - the background color of the barcode. Similar to the foreground color, it is interpreted in the the BGR format.
- \t - displays the barcode text along with the image.
- \d - add start/stop characters (valid for Code39 and NW7)
You can find more information about DisplayBarcode switches in the official documentation
If you want to create barcode during a mail merge operation you can check out our Barcodes Mail Merge example.
Creating unsupported barcodes
Not all barcode types are supported by the DisplayBarcode field in MS Word. However, you can use 3rd party libraries in combination with GemBox.Document to create documents with unsupported barcode types like PDF417.
ZXing.Net is a popular open source library that is capable of creating PDF417 images. You can add this library as a package by using the following commands in the NuGet Package Manager Console:
Install-Package ZXing.Net
Install-Package ZXing.Net.Bindings.SkiaSharp
The following example shows how to use ZXing.Net in combination with GemBox.Document to create a PDF file with a PDF417 barcode image.
using GemBox.Document;
using SkiaSharp;
using System.IO;
using ZXing;
using ZXing.Common;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a new document.
var document = new DocumentModel();
var pdf417Stream = GenerateBarcodeStream("PDF417 barcode content");
var pdf417Image = new Picture(document, pdf417Stream);
document.Sections.Add(
new Section(document,
new Paragraph(document, "PDF417:"),
new Paragraph(document, pdf417Image)));
document.Save("PDF417.pdf");
}
// Uses ZXing.NET library to save PDF417 image to memory stream.
static MemoryStream GenerateBarcodeStream(string data)
{
var writer = new ZXing.SkiaSharp.BarcodeWriter()
{
Format = BarcodeFormat.PDF_417,
Options = new EncodingOptions()
{
Width = 300,
Height = 150
}
};
var stream = new MemoryStream();
using (var bitmap = writer.Write(data))
using (var bitmapData = bitmap.Encode(SKEncodedImageFormat.Png, 100))
{
bitmapData.SaveTo(stream);
stream.Position = 0;
return stream;
}
}
}
Imports GemBox.Document
Imports SkiaSharp
Imports System.IO
Imports ZXing
Imports ZXing.Common
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a new document.
Dim document As New DocumentModel()
Dim pdf417Stream = GenerateBarcodeStream("PDF417 barcode content")
Dim pdf417Image As New Picture(document, pdf417Stream)
document.Sections.Add(
New Section(document,
New Paragraph(document, "PDF417: "),
New Paragraph(document, pdf417Image)))
document.Save("PDF417.pdf")
End Sub
' Uses ZXing.NET library to save PDF417 image to memory stream.
Function GenerateBarcodeStream(ByVal data As String) As MemoryStream
Dim writer = New ZXing.SkiaSharp.BarcodeWriter() With
{
.Format = BarcodeFormat.PDF_417,
.Options = New EncodingOptions() With
{
.Width = 300,
.Height = 150
}
}
Dim stream = New MemoryStream()
Using bitmap = writer.Write(data)
Using bitmapData = bitmap.Encode(SKEncodedImageFormat.Png, 100)
bitmapData.SaveTo(stream)
stream.Position = 0
Return stream
End Using
End Using
End Function
End Module
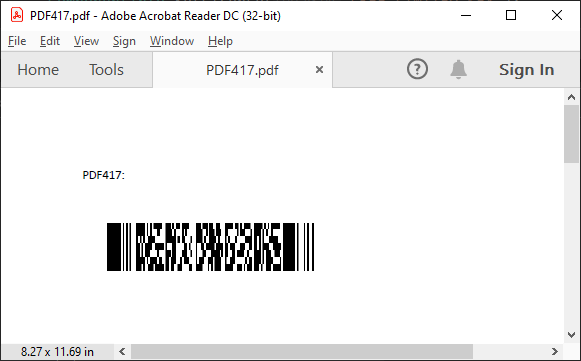