Delete Content
You can remove any element from the document by removing it from the parent element. This can be done by using the ElementCollection.RemoveAt
method on the Element.ParentCollection.
collection.
You can also delete any arbitrary document content like parts of an element, as well as single or multiple elements. This is done by using the ContentRange.Delete
method.
The following example shows how you can delete different parts of the document.
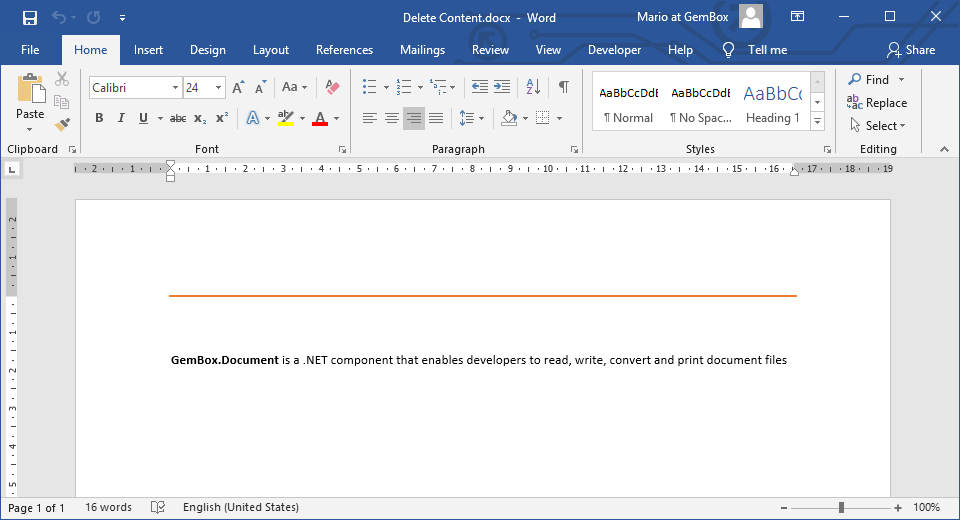
using System.Linq;
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#Reading.docx%");
// Delete 1st paragraph's inlines.
var paragraph1 = document.Sections[0].Blocks.Cast<Paragraph>(0);
paragraph1.Inlines.Content.Delete();
// Delete 3rd and 4th run from the 2nd paragraph.
var paragraph2 = document.Sections[0].Blocks.Cast<Paragraph>(1);
var runsContent = new ContentRange(
paragraph2.Inlines[2].Content.Start,
paragraph2.Inlines[3].Content.End);
runsContent.Delete();
// Delete specified text content.
var bracketContent = document.Content.Find("(").First();
bracketContent.Delete();
document.Save("Delete Content.%OutputFileType%");
}
}
Imports System.Linq
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#Reading.docx%")
' Delete 1st paragraph's inlines.
Dim paragraph1 = document.Sections(0).Blocks.Cast(Of Paragraph)(0)
paragraph1.Inlines.Content.Delete()
' Delete 3rd and 4th run from the 2nd paragraph.
Dim paragraph2 = document.Sections(0).Blocks.Cast(Of Paragraph)(1)
Dim runsContent = New ContentRange(
paragraph2.Inlines(2).Content.Start,
paragraph2.Inlines(3).Content.End)
runsContent.Delete()
' Delete specified text content.
Dim bracketContent = document.Content.Find("(").First()
bracketContent.Delete()
document.Save("Delete Content.%OutputFileType%")
End Sub
End Module