Create Word (DOCX) or PDF in Docker container
GemBox.Document can be used inside docker containers that are running .NET Docker images.
To use Docker, you must first install Docker Desktop. After that, you can containerize your .NET application.
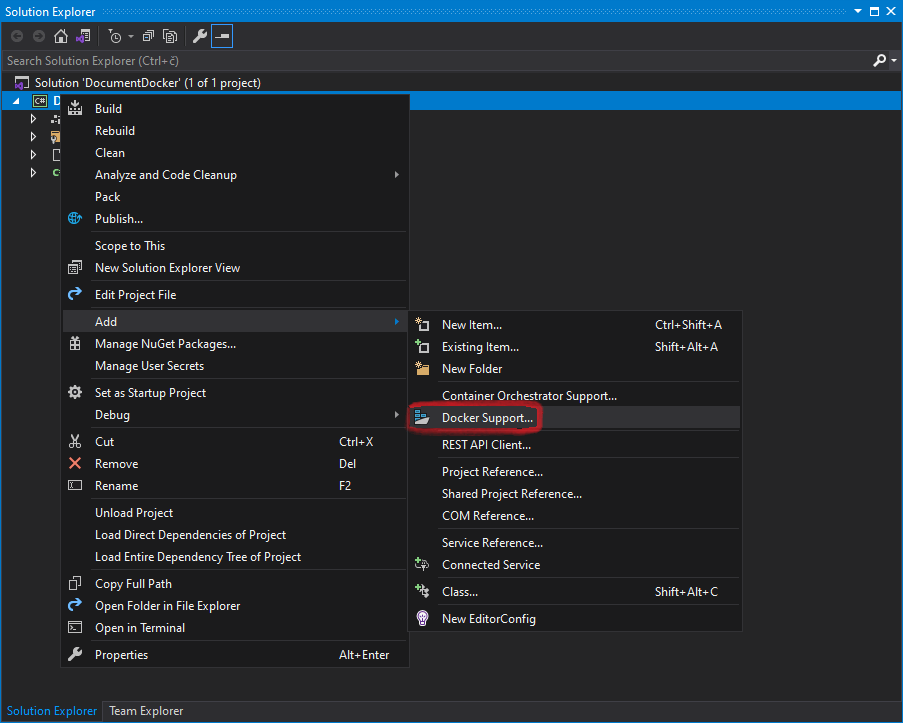
The following is a project file for the .NET Core application with added Docker support.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net8.0</TargetFramework>
<DockerDefaultTargetOS>Linux</DockerDefaultTargetOS>
<DockerfileContext>.</DockerfileContext>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Document" Version="*" />
<PackageReference Include="SkiaSharp.NativeAssets.Linux" Version="*" />
<PackageReference Include="HarfBuzzSharp.NativeAssets.Linux" Version="*" />
</ItemGroup>
</Project>
To configure or customize a Docker image, you'll need to edit the Dockerfile.
When creating PDF or image files, the font files need to be present in the container. The official Linux images for .NET won't have any fonts installed. So, you'll need to copy the necessary font files to the Linux container, or install a font package like ttf-mscorefonts-installer, or add them as custom fonts.
The following example shows how you can create DOCX and PDF files from Docker containers and configure Docker images with Dockerfile.
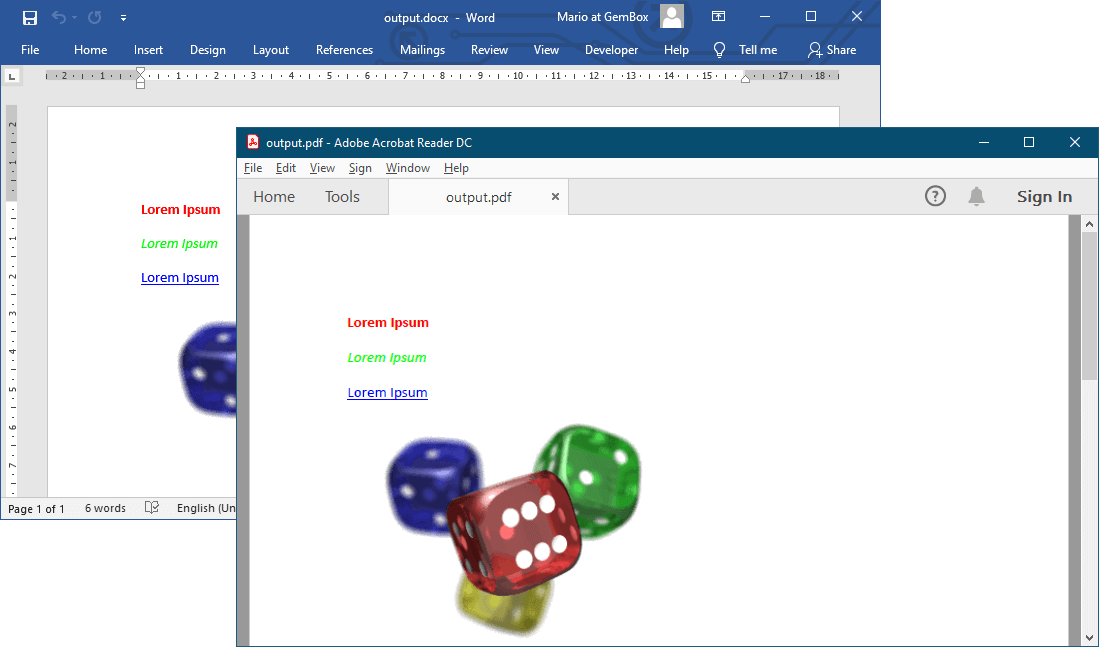
FROM mcr.microsoft.com/dotnet/runtime:8.0 AS base
WORKDIR /app
FROM mcr.microsoft.com/dotnet/sdk:8.0 AS build
WORKDIR /src
COPY ["DocumentDocker.csproj", ""]
RUN dotnet restore "./DocumentDocker.csproj"
COPY . .
WORKDIR "/src/."
RUN dotnet build "DocumentDocker.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "DocumentDocker.csproj" -c Release -o /app/publish /p:UseAppHost=false
FROM base AS final
# Update package sources to include supplemental packages (contrib archive area).
RUN sed -i 's/main/main contrib/g' /etc/apt/sources.list.d/debian.sources
# Downloads the package lists from the repositories.
RUN apt-get update
# Install font configuration.
RUN apt-get install -y fontconfig
# Install Microsoft TrueType core fonts.
RUN apt-get install -y ttf-mscorefonts-installer
# Or install Liberation TrueType fonts.
# RUN apt-get install -y fonts-liberation
# Or some other font package...
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "DocumentDocker.dll"]
using GemBox.Document;
class Program
{
static void Main()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new document.
var document = new DocumentModel();
// Add sample text.
document.Content.End
.LoadText("Lorem Ipsum\n", new CharacterFormat() { FontColor = Color.Red, Bold = true })
.LoadText("Lorem Ipsum\n", new CharacterFormat() { FontColor = Color.Green, Italic = true })
.LoadText("Lorem Ipsum\n", new CharacterFormat() { FontColor = Color.Blue, UnderlineStyle = UnderlineType.Single });
// Add sample image.
document.Content.End
.InsertRange(new Picture(document, "%#Dices.png%").Content);
// Save document in DOCX and PDF format.
document.Save("output.docx");
document.Save("output.pdf");
}
}
Imports GemBox.Document
Module Program
Sub Main()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new document.
Dim document As New DocumentModel()
' Add sample text.
document.Content.End _
.LoadText("Lorem Ipsum" + vbLf, New CharacterFormat() With {.FontColor = Color.Red, .Bold = True}) _
.LoadText("Lorem Ipsum" + vbLf, New CharacterFormat() With {.FontColor = Color.Green, .Italic = True}) _
.LoadText("Lorem Ipsum" + vbLf, New CharacterFormat() With {.FontColor = Color.Blue, .UnderlineStyle = UnderlineType.Single})
' Add sample image.
document.Content.End _
.InsertRange(New Picture(document, "%#Dices.png%").Content)
' Save document in DOCX and PDF format.
document.Save("output.docx")
document.Save("output.pdf")
End Sub
End Module
Using full functionality of GemBox.Document on a Linux host requires adjustments explained in detail on Supported Platforms help page.