Create Content Controls in Word Files
The following example shows how you can create various Content Controls and change their properties using the GemBox.Document component in C# and VB.NET.
using GemBox.Document;
using GemBox.Document.CustomMarkups;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var section = new Section(document);
document.Sections.Add(section);
// Create locked Rich Text Content Control.
var richTextControl = new BlockContentControl(document, ContentControlType.RichText,
new Paragraph(document, "This text is inside Rich Text Content Control."),
new Paragraph(document, "It cannot be deleted or edited."));
richTextControl.Properties.LockEditing = true;
richTextControl.Properties.LockDeleting = true;
section.Blocks.Add(richTextControl);
// Create named Plain Text Content Control.
var plainTextControl = new BlockContentControl(document, ContentControlType.PlainText,
new Paragraph(document, "Plain Text Content Control with tag and title."));
plainTextControl.Properties.Tag = "Plain Text Name";
plainTextControl.Properties.Title = "Plain Text Title";
section.Blocks.Add(plainTextControl);
// Create CheckBox Content Control.
var checkBoxControl = new InlineContentControl(document, ContentControlType.CheckBox);
checkBoxControl.Properties.CharacterFormat = new CharacterFormat() { FontName = "MS Gothic" };
checkBoxControl.Properties.Checked = true;
// Create ComboBox Content Control.
var comboBoxControl = new InlineContentControl(document, ContentControlType.ComboBox,
new Run(document, "<Select GemBox Component>"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("<Select GemBox Component>", "NONE"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Spreadsheet", "GBS"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Document", "GBD"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Pdf", "GBA"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Presentation", "GBP"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Email", "GBE"));
comboBoxControl.Properties.ListItems.Add(new ContentControlListItem("GemBox.Imaging", "GBI"));
section.Blocks.Add(new Paragraph(document,
checkBoxControl,
new SpecialCharacter(document, SpecialCharacterType.LineBreak),
comboBoxControl));
document.Save("Content Controls.docx");
}
}
Imports GemBox.Document
Imports GemBox.Document.CustomMarkups
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim section As New Section(document)
document.Sections.Add(section)
' Create locked Rich Text Content Control.
Dim richTextControl As New BlockContentControl(document, ContentControlType.RichText,
New Paragraph(document, "This text is inside Rich Text Content Control."),
New Paragraph(document, "It cannot be deleted or edited."))
richTextControl.Properties.LockEditing = True
richTextControl.Properties.LockDeleting = True
section.Blocks.Add(richTextControl)
' Create named Plain Text Content Control.
Dim plainTextControl As New BlockContentControl(document, ContentControlType.PlainText,
New Paragraph(document, "Plain Text Content Control with tag and title."))
plainTextControl.Properties.Tag = "Plain Text Name"
plainTextControl.Properties.Title = "Plain Text Title"
section.Blocks.Add(plainTextControl)
' Create CheckBox Content Control.
Dim checkBoxControl As New InlineContentControl(document, ContentControlType.CheckBox)
checkBoxControl.Properties.CharacterFormat = New CharacterFormat() With {.FontName = "MS Gothic"}
checkBoxControl.Properties.Checked = True
' Create ComboBox Content Control.
Dim comboBoxControl As New InlineContentControl(document, ContentControlType.ComboBox,
New Run(document, "<Select GemBox Component>"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("<Select GemBox Component>", "NONE"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Spreadsheet", "GBS"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Document", "GBD"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Pdf", "GBA"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Presentation", "GBP"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Email", "GBE"))
comboBoxControl.Properties.ListItems.Add(New ContentControlListItem("GemBox.Imaging", "GBI"))
section.Blocks.Add(New Paragraph(document,
checkBoxControl,
New SpecialCharacter(document, SpecialCharacterType.LineBreak),
comboBoxControl))
document.Save("Content Controls.docx")
End Sub
End Module
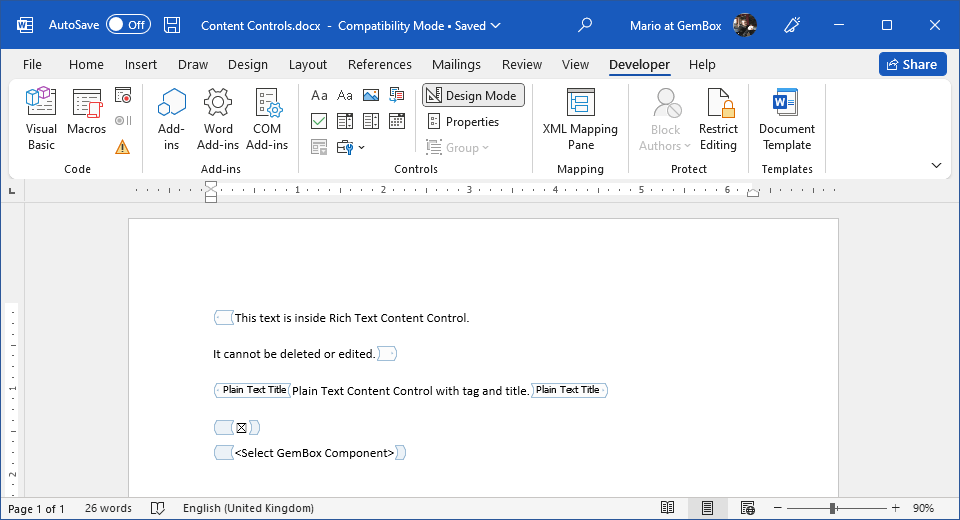
The The following example shows how you can update Content Controls with values from mapped With GemBox.Document you can update the values separately in mapped XML ( The following example shows how you can update the ContentControlType
enumeration contains a list of Content Control types supported through GemBox.Document's API. Each Content Control element can be customized using the ContentControlProperties
class.Content Controls with XML Mapping
CustomXmlPart
using the IContentControl.Update
method.using GemBox.Document;
using GemBox.Document.CustomMarkups;
using System;
using System.Linq;
using System.Text;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#XmlMapping.docx%");
// Edit mapped XML part.
document.CustomXmlParts[0].Data = Encoding.UTF8.GetBytes(
@"<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<customer>
<firstName>Jane</firstName>
<lastName>Doe</lastName>
<birthday>2010-01-01T00:00:00</birthday>
<married>true</married>
</customer>");
// Update Content Controls inlines or blocks based on the values from mapped XML part.
foreach (var contentControl in document.GetChildElements(true).OfType<IContentControl>())
contentControl.Update();
document.Save("Updated ContentControls.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.CustomMarkups
Imports System
Imports System.Linq
Imports System.Text
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#XmlMapping.docx%")
' Edit mapped XML part.
document.CustomXmlParts(0).Data = Encoding.UTF8.GetBytes(
"<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<customer>
<firstName>Jane</firstName>
<lastName>Doe</lastName>
<birthday>2010-01-01T00:00:00</birthday>
<married>true</married>
</customer>")
' Update Content Controls inlines or blocks based on the values from mapped XML part.
For Each contentControl In document.GetChildElements(True).OfType(Of IContentControl)()
contentControl.Update()
Next
document.Save("Updated ContentControls.%OutputFileType%")
End Sub
End Module
CustomXmlPart.Data
) and the content of Content Control (BlockContentControl.Blocks
or InlineContentControl.Inlines
).CustomXmlPart
source with values from the mapped Content Controls elements using the IContentControl.UpdateSource
method.using GemBox.Document;
using GemBox.Document.CustomMarkups;
using System;
using System.Linq;
using System.Text;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#XmlMapping.docx%");
// Edit Content Controls.
foreach (var contentControl in document.GetChildElements(true).OfType<IContentControl>())
{
switch (contentControl.Properties.Title)
{
case "FirstName":
contentControl.Content.LoadText("Joe");
break;
case "LastName":
contentControl.Content.LoadText("Smith");
break;
case "Birthday":
contentControl.Properties.Date = new DateTime(2002, 2, 2);
break;
case "Married":
contentControl.Properties.Checked = true;
break;
}
// Update mapped XML part based on the content from Content Control.
contentControl.UpdateSource();
}
document.Save("Updated XmlMapping.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.CustomMarkups
Imports System
Imports System.Linq
Imports System.Text
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#XmlMapping.docx%")
' Edit Content Controls.
For Each contentControl In document.GetChildElements(True).OfType(Of IContentControl)()
Select Case contentControl.Properties.Title
Case "FirstName"
contentControl.Content.LoadText("Joe")
Case "LastName"
contentControl.Content.LoadText("Smith")
Case "Birthday"
contentControl.Properties.Date = New DateTime(2002, 2, 2)
Case "Married"
contentControl.Properties.Checked = True
End Select
' Update mapped XML part based on the content from Content Control.
contentControl.UpdateSource()
Next
document.Save("Updated XmlMapping.%OutputFileType%")
End Sub
End Module