Getting started with GemBox.Document
GemBox.Document is a .NET component that enables you to read, write, convert, and print document files (DOCX, DOC, PDF, RTF, HTML, and ODT) from .NET applications. GemBox.Document requires only .NET. It supports a wide range of .NET versions, as listed below: Before you can use GemBox.Document, you need to install it in your project. The best way is by adding a GemBox.Document NuGet package via NuGet Package Manager in Visual Studio. To install the package, you can follow the steps listed below. However, please note that this guide assumes you have already set up a Visual Studio project. If you haven't done that yet, you can refer to the official tutorial for guidance. As an alternative, you can open the NuGet Package Manager Console (Tools -> NuGet Package Manager -> Package Manager Console) and run the following command: We also provide DLL and setup downloads on GemBox.Document's BugFixes page. Setup download links are at the bottom of the page, under the 'Global Assembly' section. Once you have installed GemBox.Document, all you have to do is add the using directive and make sure you call the The ComponentInfo.SetLicense requires a license key as a parameter. If you are using the component in a Free mode, use "FREE-LIMITED-KEY" as a license key, and if you have purchased a Professional license, use the key you got in your email after the purchase. You can read more about GemBox.Document's working modes on the Evaluation and Licensing page. After that, you can write your application code for working with documents, like the code below. It shows how to create a simple Word document using GemBox.Document's content model and populate it using common document elements like You can click the 'Run Example' button to run the code and download the resulting file. You can also change the output format by selecting a corresponding file type from the 'Output file type' dropdown control.Requirements
Installation
Install-Package GemBox.Document
Usage
ComponentInfo.SetLicense
method before using any member of the component. We suggest putting the call at the beginning of the Main()
method.Section
, Paragraph
and Run
, and then save the DocumentModel
to a DOCX file.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
DocumentModel document = new DocumentModel();
Section section = new Section(document);
document.Sections.Add(section);
Paragraph paragraph = new Paragraph(document);
section.Blocks.Add(paragraph);
Run run = new Run(document, "Hello World!");
paragraph.Inlines.Add(run);
document.Save("HelloWorld.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim section As New Section(document)
document.Sections.Add(section)
Dim paragraph As New Paragraph(document)
section.Blocks.Add(paragraph)
Dim run As New Run(document, "Hello World!")
paragraph.Inlines.Add(run)
document.Save("HelloWorld.%OutputFileType%")
End Sub
End Module
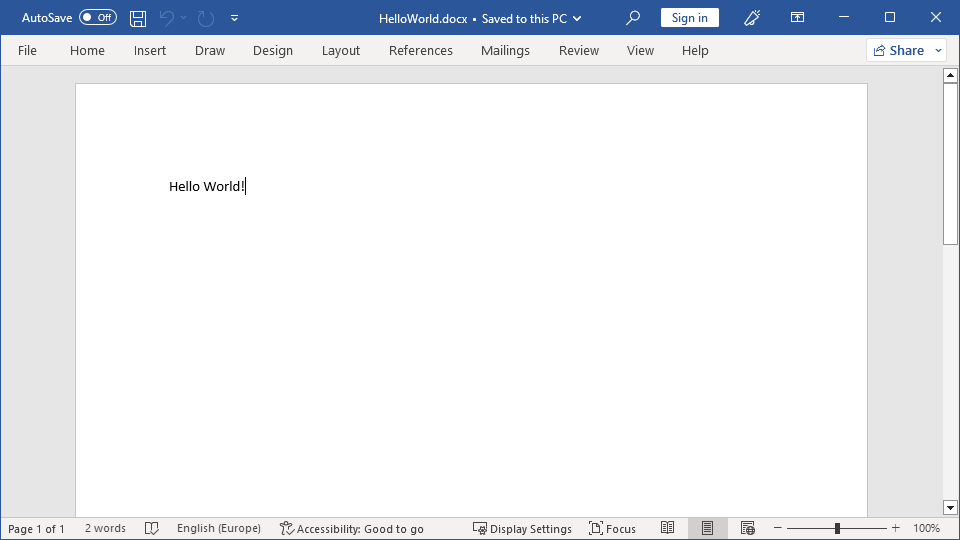
To learn more about GemBox.Document, check out our collection of examples listed on the menu. These examples will show you how to use the component in various document-processing tasks and platforms. Additionally, for detailed information on the API, head to our documentation pages. This resource offers a comprehensive API reference, explaining each method and property available within the component.