Bookmarks and Hyperlinks
The following example shows how you can use the GemBox.Document component to insert a bookmark into a document and add a hyperlink that links to that bookmark.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var bookmarkName = "TopOfDocument";
document.Sections.Add(
new Section(document,
new Paragraph(document,
new BookmarkStart(document, bookmarkName),
new Run(document, "This is a 'TopOfDocument' bookmark."),
new BookmarkEnd(document, bookmarkName)),
new Paragraph(document,
new Run(document, "The following is a link to "),
new Hyperlink(document, "https://www.gemboxsoftware.com/document", "GemBox.Document Overview"),
new Run(document, " page.")),
new Paragraph(document,
new SpecialCharacter(document, SpecialCharacterType.PageBreak),
new Run(document, "This is a document's second page."),
new SpecialCharacter(document, SpecialCharacterType.LineBreak),
new Hyperlink(document, bookmarkName, "Return to 'TopOfDocument'.") { IsBookmarkLink = true })));
document.Save("Bookmarks and Hyperlinks.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim bookmarkName = "TopOfDocument"
document.Sections.Add(
New Section(document,
New Paragraph(document,
New BookmarkStart(document, bookmarkName),
New Run(document, "This is a 'TopOfDocument' bookmark."),
New BookmarkEnd(document, bookmarkName)),
New Paragraph(document,
New Run(document, "The following is a link to "),
New Hyperlink(document, "https://www.gemboxsoftware.com/document", "GemBox.Document Overview"),
New Run(document, " page.")),
New Paragraph(document,
New SpecialCharacter(document, SpecialCharacterType.PageBreak),
New Run(document, "This is a document's second page."),
New SpecialCharacter(document, SpecialCharacterType.LineBreak),
New Hyperlink(document, bookmarkName, "Return to 'TopOfDocument'.") With {.IsBookmarkLink = True})))
document.Save("Bookmarks and Hyperlinks.%OutputFileType%")
End Sub
End Module
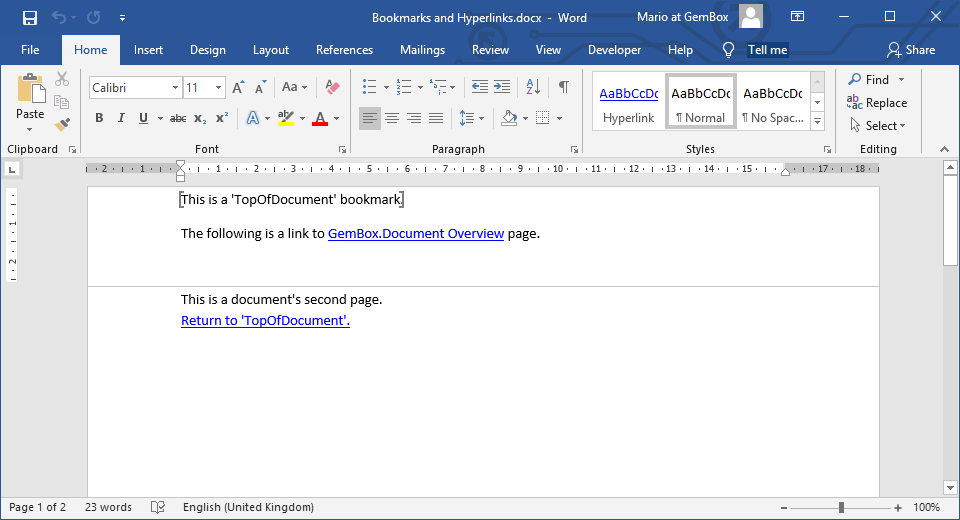
You can use the DocumentModel.Bookmarks
collection to iterate through all bookmarks in the document, or to remove any bookmark from the document. You can also use it to retrieve a single Bookmark
by specifying the bookmark's name.
When converting a Word document to PDF file, you can specify if the document's bookmarks should be exported as PDF outlines using the The following example shows how you can use GemBox.Document to modify existing bookmarks by replacing their content with text, table, and image. With the Note, if your require modifying or replacing the bookmark's content in a repeated way, like inserting a dynamic number of table rows, than a better option would be to use Mail Merge, a powerful and customizable process of merging or importing data from a data source to a template document. Besides modifying the bookmark's content, you can also retrieve the bookmark's text using the PdfSaveOptions.BookmarksCreateOptions
property.Modify Word Bookmarks
using GemBox.Document;
using GemBox.Document.Tables;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#BookmarksTemplate.docx%");
// Replace bookmark's content with plain text.
document.Bookmarks["Company"].GetContent(false).LoadText("Acme Corporation");
// Replace bookmark's content with HTML text.
document.Bookmarks["Address"].GetContent(false).LoadText(
"<span style='font: italic 8pt Calibri; color: red;'>240 Old Country Road, Springfield, IL</span>",
LoadOptions.HtmlDefault);
// Insert hyperlink into a bookmark.
var hyperlink = new Hyperlink(document, "mailto:joe.doe@acme.co", "joe.doe@acme.co");
document.Bookmarks["Contact"].GetContent(false).Set(hyperlink.Content);
// Insert image into a bookmark.
var picture = new Picture(document, "%#Acme.png%");
document.Bookmarks["Logo"].GetContent(false).Set(picture.Content);
// Insert text and table into a bookmark.
ContentRange itemsRange = document.Bookmarks["Items"].GetContent(false);
itemsRange = itemsRange.LoadText("Storage:");
var table = new Table(document, 6, 3, (r, c) => new TableCell(document, new Paragraph(document, $"Item {r}-{c}")));
itemsRange.End.InsertRange(table.Content);
document.Save("Modified Bookmarks.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Tables
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#BookmarksTemplate.docx%")
' Replace bookmark's content with plain text.
document.Bookmarks("Company").GetContent(False).LoadText("Acme Corporation")
' Replace bookmark's content with HTML text.
document.Bookmarks("Address").GetContent(False).LoadText(
"<span style='font: italic 8pt Calibri; color: red;'>240 Old Country Road, Springfield, IL</span>",
LoadOptions.HtmlDefault)
' Insert hyperlink into a bookmark.
Dim hyperlink As New Hyperlink(document, "mailto:joe.doe@acme.co", "joe.doe@acme.co")
document.Bookmarks("Contact").GetContent(False).Set(hyperlink.Content)
' Insert image into a bookmark.
Dim picture As New Picture(document, "%#Acme.png%")
document.Bookmarks("Logo").GetContent(False).Set(picture.Content)
' Insert text and table into a bookmark.
Dim itemsRange As ContentRange = document.Bookmarks("Items").GetContent(False)
itemsRange = itemsRange.LoadText("Storage:")
Dim table As New Table(document, 6, 3, Function(r, c) New TableCell(document, New Paragraph(document, $"Item {r}-{c}")))
itemsRange.End.InsertRange(table.Content)
document.Save("Modified Bookmarks.%OutputFileType%")
End Sub
End Module
Bookmark.GetContent
method you can retrieve the bookmark's content as a ContentRange
object which you can use to delete or replace that content.ContentRange.ToString
method, or retrieve other elements in the bookmark, like tables and images, using the ContentRange.GetChildElements
method.