Create or Generate PDF files in C# and VB.NET
While handling office files, it's sometimes necessary to generate PDF files that offer portability, editability, compatibility, and quality.
With GemBox.Document you can create PDF files from .NET applications simply and efficiently without using Microsoft Word on either developer or client machines.
This article will guide you through the process of generating PDFs using GemBox.Document in C# and VB.NET. With short code samples, you will see how you can insert text, images, and tables into your PDF files and use encryption and digital signatures on them.
The article will cover the following topics:
- Install and configure the GemBox.Document library
- How to easily generate a PDF file in C#
- How to insert images and tables to a PDF file in C#
Install and configure the GemBox.Document library
The first step before generating a PDF file is creating a new .NET project. If you are still getting familiar with Visual Studio or need a reminder, refer to the official Microsoft tutorial. Also, although GemBox.Document supports a wide range of .NET versions (from .NET Framework 4.6.2), we recommend using the newest version.
Then you need to install the GemBox.Document component, and the fastest way of doing that is via NuGet Package Manager.
- In the Solution Explorer window, right-click on the solution and select 'Manage NuGet Packages for Solution'.
- Search for GemBox.Document and click on 'Install'.
Alternatively, you can open the NuGet Package Manager Console (Tools -> NuGet Package Manager -> Package Manager Console) and run the following command:
Install-Package GemBox.Document
Now that you have installed the GemBox.Document library, all you have to do is make sure you call the ComponentInfo.SetLicense method before using any other member of the library.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
Since we are working with a console application, we suggest putting this line at the beginning of the main()
method.
In this tutorial, we will work in the free mode, which allows you to use the library without purchasing the license, but with limitations. You can read more about working modes and limitations on the Evaluation and Licensing page.
How to easily generate a PDF file in C#
The following code snippets show how you can create a simple document file from scratch and save it as a PDF using GemBox.Document.
- First, create a new empty document.
- Next, you will add the text “Hello Word!”.
- Finally, save the generated document as a PDF file.
Refer to the following image for the result of the previous code.
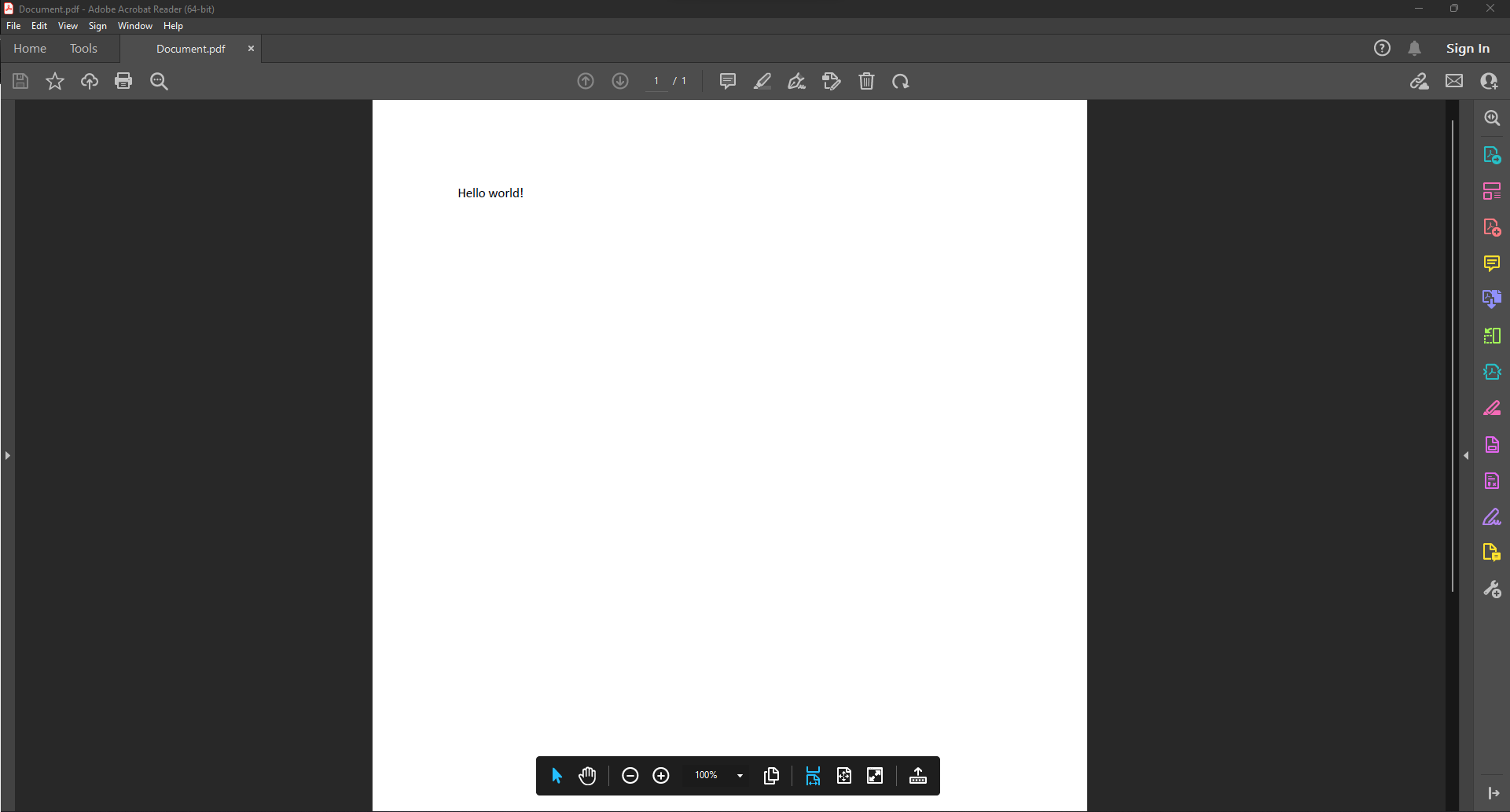
How to insert images and tables into a PDF file
This section will teach you how to create PDF files with more advanced content, such as images and tables.
- First, create a new empty document, and an empty section.
- Then insert an image using the
new picture()
method. In this case, you will set its size to 100x100 points and add it to the section.var picture = new Picture(document, "picture path"); picture.Layout = Layout.Inline(new Size(100, 100)); section.Blocks.Add(new Paragraph(document, picture));
Dim Picture = New Picture(document, "picture path") Picture.Layout = Layout.Inline(New Size(100, 100)) Section.Blocks.Add(New Paragraph(document, Picture))
- Next, you will create a 3x5 table, format it, and add it to the section.
var table = new Table(document, 3, 5); table.TableFormat.Borders.SetBorders(MultipleBorderTypes.All, BorderStyle.Dashed, Color.Blue, 1); table.TableFormat.PreferredWidth = new TableWidth(100, TableWidthUnit.Percentage); section.Blocks.Add(table);
Dim Table = New Table(document, 3, 5) Table.TableFormat.Borders.SetBorders(MultipleBorderTypes.All, BorderStyle.Dashed, Color.Blue, 1) Table.TableFormat.PreferredWidth = New TableWidth(100, TableWidthUnit.Percentage) Section.Blocks.Add(Table)
- Finally, save the generated document as a PDF file.
Refer to the following image for the result of the previous code.
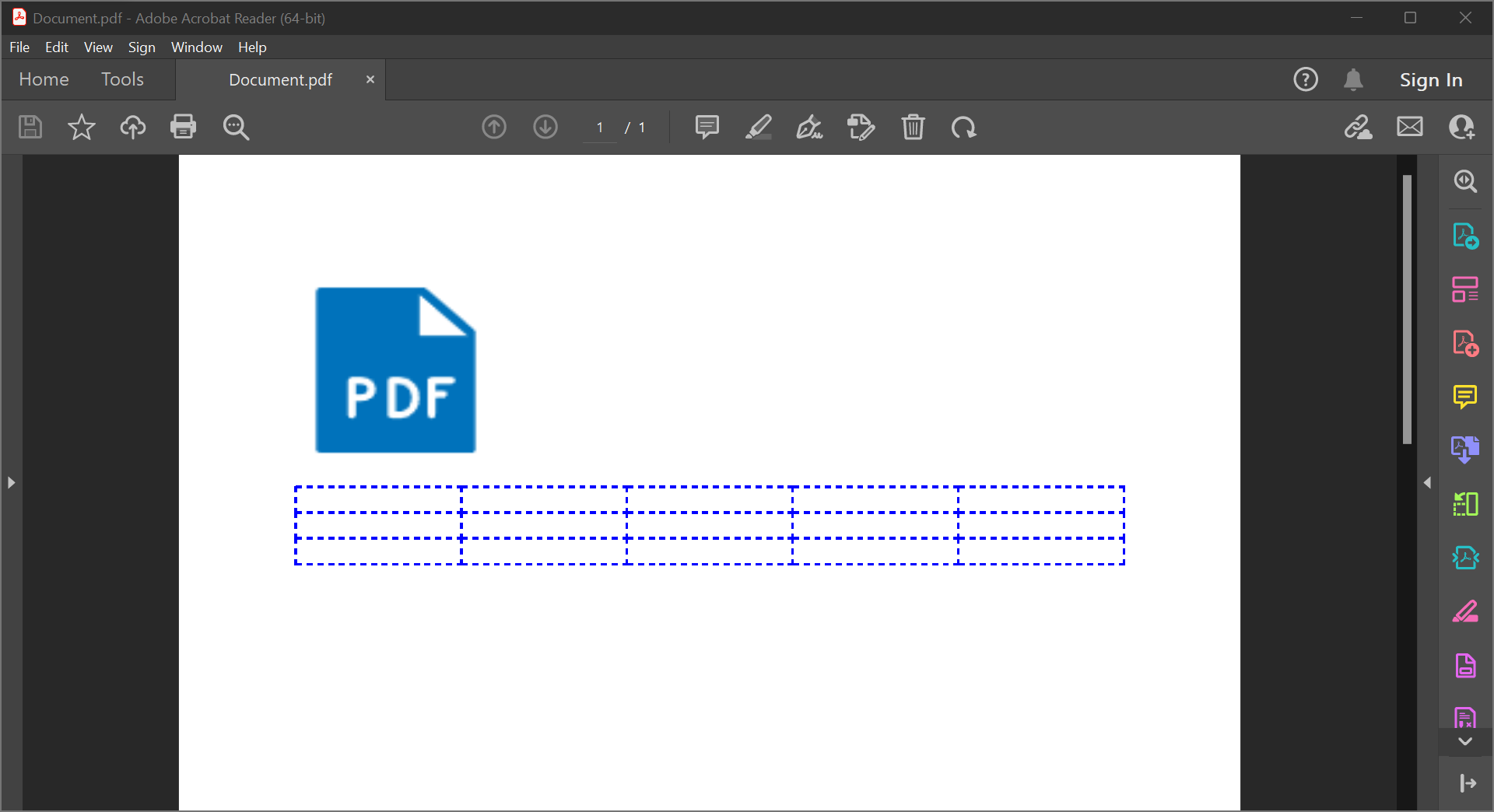
Conclusion
In this article, you learned how to create PDF files from scratch using C# and VB.NET. You can read more about support for PDF with the GemBox.Document API on the documentation page.
You can explore theGemBox.PDF component, which focuses on executing low-level object manipulations, reading, writing, merging, and splitting PDF files from .NET applications.