How to convert Word (DOCX) to PDF using C# and VB.NET
Maintaining the same formatting and giving a shareable aspect to important documents are the main reasons why we often need to convert Word documents to PDF files.
You need to use the fastest solution possible when you are handling several DOCX files programmatically and need to convert them to PDF.
With GemBox.Document you can convert word files to PDF in C# and VB.NET without using Microsoft Office Interop. GemBox.Document's API is simpler and more intuitive so you will be able to save hours of programming.
This article covers the following topics:
How to install and configure the GemBox.Document library
Before you start, you need to install GemBox.Document. The best way to do that is to install the NuGet Package by following these instructions:
Add the GemBox.Document component as a package using the following command from the NuGet Package Manager Console:
Install-Package GemBox.Document
After installing the GemBox.Document library, you must call the ComponentInfo.SetLicense method before using any other member of the library.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
In this tutorial, by using “FREE-LIMITED-KEY”, you will be using GemBox's free mode. This mode allows you to use the library without purchasing a license, but with some limitations. If you purchased a license, you can replace “FREE-LIMITED-KEY” with your serial key.
You can check this page for a complete step-by-step guide to installing and setting up GemBox.Document in other ways.
How to convert Word (DOCX) files to PDF in C#
If you want to convert a Word file to PDF, you just need to follow these two simple steps below:
- Load the DOCX file into the
DocumentModel
object.var document = DocumentModel.Load("Input.docx");
- Then save the DocumentModel object to a PDF file.
document.Save("Output.pdf");
This can can be simplified even more by writing it in a single line of code:
DocumentModel.Load("Input.docx").Save("Output.pdf");
Note that when you convert a DOCX file to PDF in C#, the machine that's executing the code should have the fonts used in the document installed. If it doesn't, you can provide them as files or as embedded resources. |
The following screenshots show how the output file will look like:
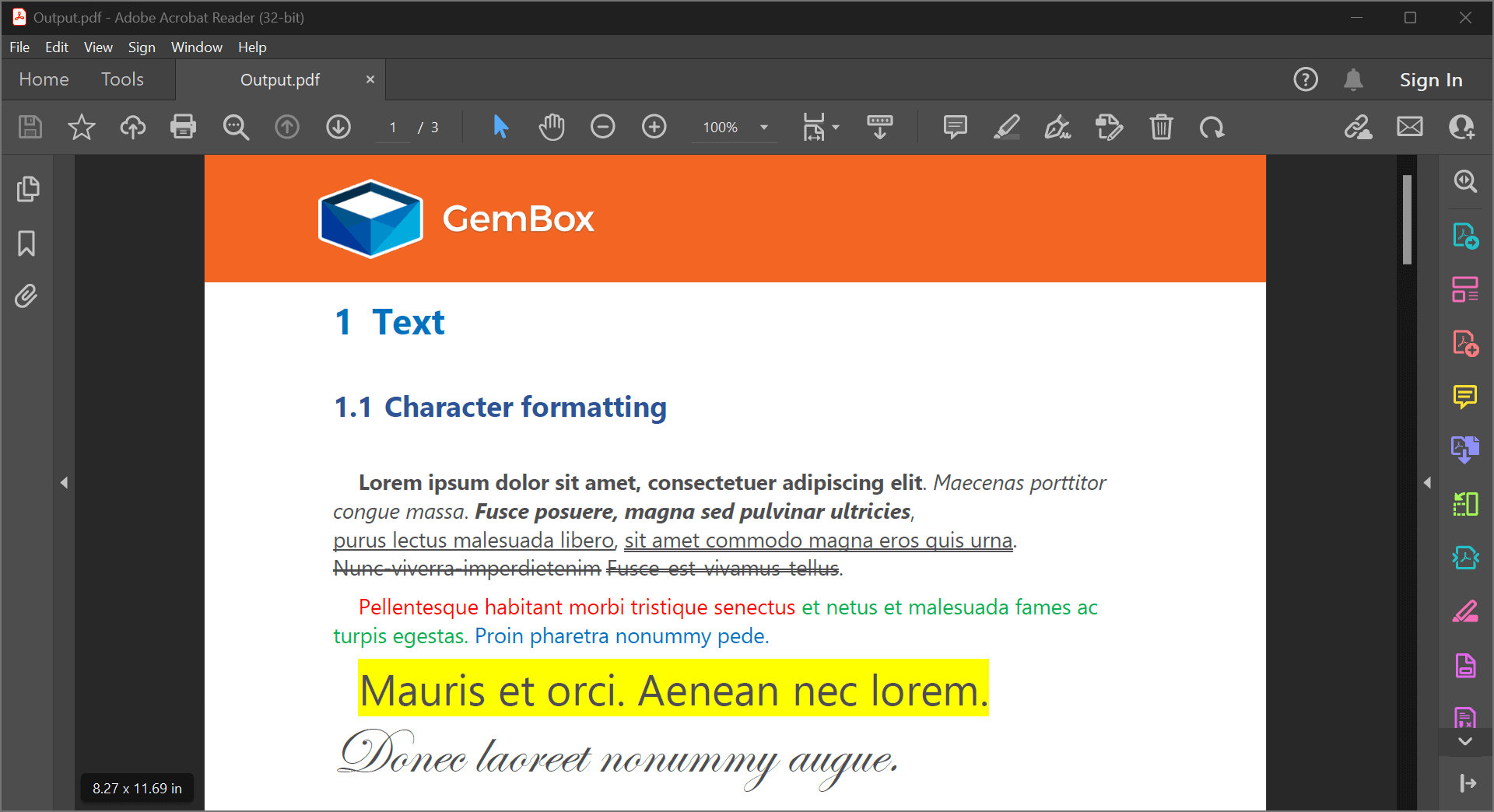
How to convert Word pages to PDF files
Besides converting a whole Word document to a single PDF file, it's also possible to convert each page of a document into a separate PDF file.
To perform this type of DOCX page to PDF conversion, you can follow these steps:
- Load the DOCX file you need to convert to PDF using the DocumentModel.Load() method.
var document = DocumentModel.Load("Input.docx");
Get the Word pages from the document.
var pages = document.GetPaginator().Pages;
Create a
PdfSaveOptions
instance and use thePdfSaveOptions.ImageDpi
property to optimize and reduce the size of the resulting PDF files.var pdfSaveOptions = new PdfSaveOptions() { ImageDpi = 220 };
Then iterate through Word pages and save each one as a separate PDF file.
for (int pageIndex = 0; pageIndex < pages.Count; pageIndex++) { var page = pages[pageIndex]; page.Save($"Page{pageIndex}.pdf", pdfSaveOptions); }
After executing the code above, the output document should look like this:
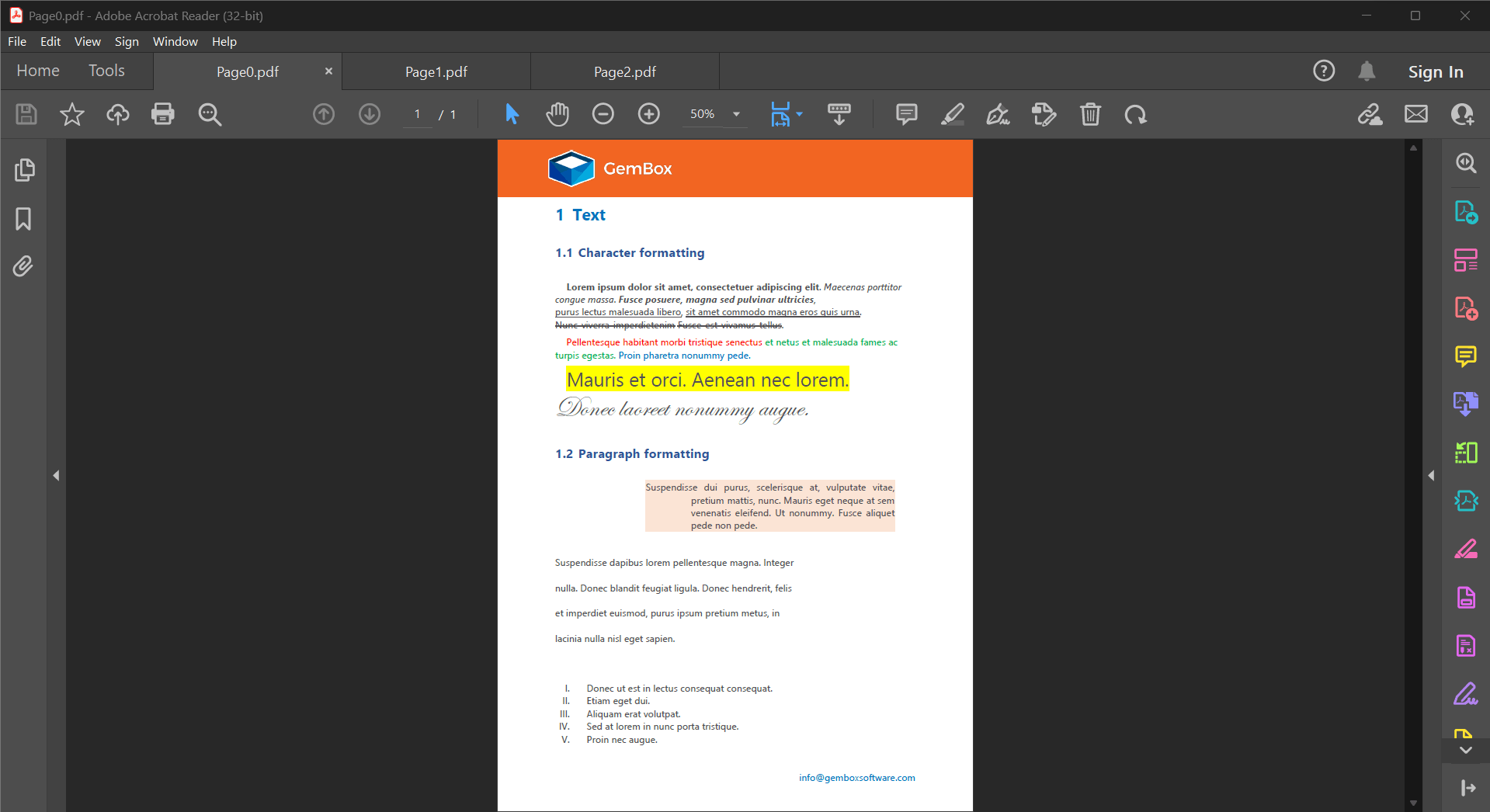
How to convert DOCX files to PDF/A in C#
GemBox.Document also supports saving the Word document to PDF/A, an ISO-standardized version of the Portable Document Format (PDF) specialized for use in archiving and long-term preservation of electronic documents.
The following example shows how you can convert a Word file to PDF/A using PdfConformanceLevel to specify the conformance level and version.
- Load the Word file using the
DocumentModel.Load()
method.var document = DocumentModel.Load("Input.docx");
- Create an instance of PdfSaveOptions and set the
PdfConformanceLevel
to PdfA1a.var options = new PdfSaveOptions() { ConformanceLevel = PdfConformanceLevel.PdfA1a };
- Finally, save the document to a PDF file.
document.Save("Output.pdf", options);
Conclusion
This article showed you how you can use GemBox.Document to convert Word to Pdf programmatically in .NET.
If you would like to learn about various ways you can convert a Pdf document to a DOCX file, check this example:
Convert PDF to Word (DOCX) in C# and VB.NET
For more information regarding the GemBox.Document API, check the documentation pages. We also recommend checking our GemBox.Document examples where you can learn how to use other features by running code.