Convert Word files to PDF
With GemBox.Document you can easily convert Word files from one format to another, using just C# or VB.NET code. You can load or read any supported input file format and save or write it as any supported output file format. The complete list of formats is available on the Supported File Formats help page.
For both reading and writing, you can either provide the file's path or a stream by using one of the DocumentModel.Load
or DocumentModel.Save
methods.
The following example shows how you can convert a Word file to PDF using the default DocxLoadOptions
and PdfSaveOptions
.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// In order to convert Word to PDF, we just need to:
// 1. Load DOC or DOCX file into DocumentModel object.
// 2. Save DocumentModel object to PDF file.
DocumentModel document = DocumentModel.Load("%InputFileName%");
document.Save("Output.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' In order to convert Word to PDF, we just need to:
' 1. Load DOC or DOCX file into DocumentModel object.
' 2. Save DocumentModel object to PDF file.
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
document.Save("Output.%OutputFileType%")
End Sub
End Module
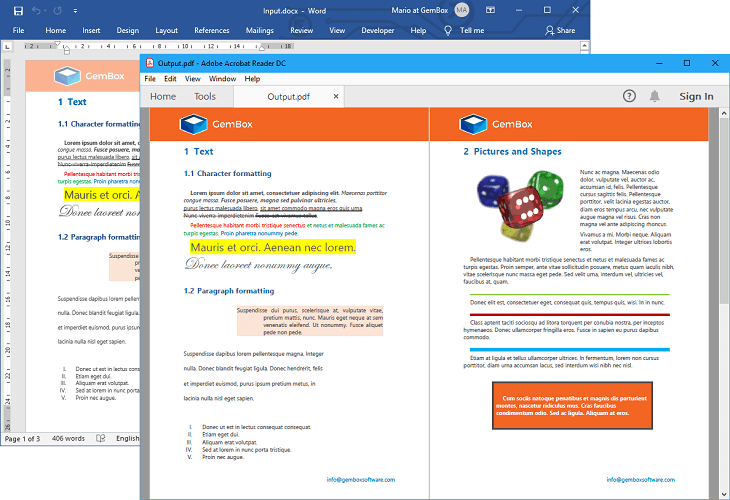
Note, when converting a Word document to PDF, the machine that's executing the code should have the fonts that are used in the document installed on it. If not, you can provide them as custom or embedded fonts. In addition to saving the whole Word document as a single PDF file, you can also convert each document's page into a separate PDF file. The following example shows how to do that, and also how to use the GemBox.Document also supports saving the Word document to PDF/A, an ISO-standardized version of the Portable Document Format (PDF) specialized for use in the archiving and long-term preservation of electronic documents. The following example shows how you can convert a Word file to PDF/A using Convert Word pages to PDF
PdfSaveOptions.ImageDpi
property to optimize and reduce the resulting PDF file sizes.using GemBox.Document;
using System.IO;
using System.IO.Compression;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load Word file.
DocumentModel document = DocumentModel.Load("%InputFileName%");
// Get Word pages.
var pages = document.GetPaginator().Pages;
// Create PDF save options.
var pdfSaveOptions = new PdfSaveOptions() { ImageDpi = 220 };
// Create ZIP file for storing PDF files.
using (var archiveStream = File.OpenWrite("Output.zip"))
using (var archive = new ZipArchive(archiveStream, ZipArchiveMode.Create))
// Iterate through Word pages.
for (int pageIndex = 0; pageIndex < pages.Count; pageIndex++)
{
DocumentModelPage page = pages[pageIndex];
// Create ZIP entry for each document page.
var entry = archive.CreateEntry($"Page {pageIndex + 1}.pdf");
// Save each document page as PDF to ZIP entry.
using (var pdfStream = new MemoryStream())
using (var entryStream = entry.Open())
{
page.Save(pdfStream, pdfSaveOptions);
pdfStream.CopyTo(entryStream);
}
}
}
}
Imports GemBox.Document
Imports System.IO
Imports System.IO.Compression
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load Word file.
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
' Get Word pages.
Dim pages = document.GetPaginator().Pages
' Create PDF save options.
Dim pdfSaveOptions As New PdfSaveOptions() With {.ImageDpi = 220}
' Create ZIP file for storing PDF files.
Using archiveStream = File.OpenWrite("Output.zip")
Using archive As New ZipArchive(archiveStream, ZipArchiveMode.Create)
' Iterate through Word pages.
For pageIndex As Integer = 0 To pages.Count - 1
Dim page As DocumentModelPage = pages(pageIndex)
' Create ZIP entry for each document page.
Dim entry = archive.CreateEntry($"Page {pageIndex + 1}.pdf")
' Save each document page as PDF to ZIP entry.
Using pdfStream As New MemoryStream()
Using entryStream = entry.Open()
page.Save(pdfStream, pdfSaveOptions)
pdfStream.CopyTo(entryStream)
End Using
End Using
Next
End Using
End Using
End Sub
End Module
Convert Word files to PDF/A
PdfConformanceLevel
to specify the conformance level and version.using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
PdfConformanceLevel conformanceLevel = %PdfConformanceLevel%;
// Load Word file.
DocumentModel document = DocumentModel.Load("%InputFileName%");
// Create PDF save options.
var options = new PdfSaveOptions()
{
ConformanceLevel = conformanceLevel
};
// Save to PDF file.
document.Save("OutputWithConformance.pdf", options);
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim conformanceLevel As PdfConformanceLevel = %PdfConformanceLevel%
' Load Word file.
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
' Create PDF save options.
Dim options As New PdfSaveOptions() With
{
.ConformanceLevel = conformanceLevel
}
' Save to PDF file.
document.Save("OutputWithConformance.pdf", options)
End Sub
End Module