VBA Macros in PowerPoint
Visual Basic for Applications is human-readable and editable programming code that gets generated when you record a macro. Today, it is widely-used with other Microsoft Office applications such as MS-Word, MS-PowerPoint, and MS-Access.
The VBA Project is where the modules and forms are stored when you write VBA code in PowerPoint.
In GemBox.Presentation vba project is represented by VbaProject
element and can be loaded from and saved to macro-enabled (PPTM) file format only.
The following example shows how you can create an PowerPoint VBA module in C# and VB.NET.
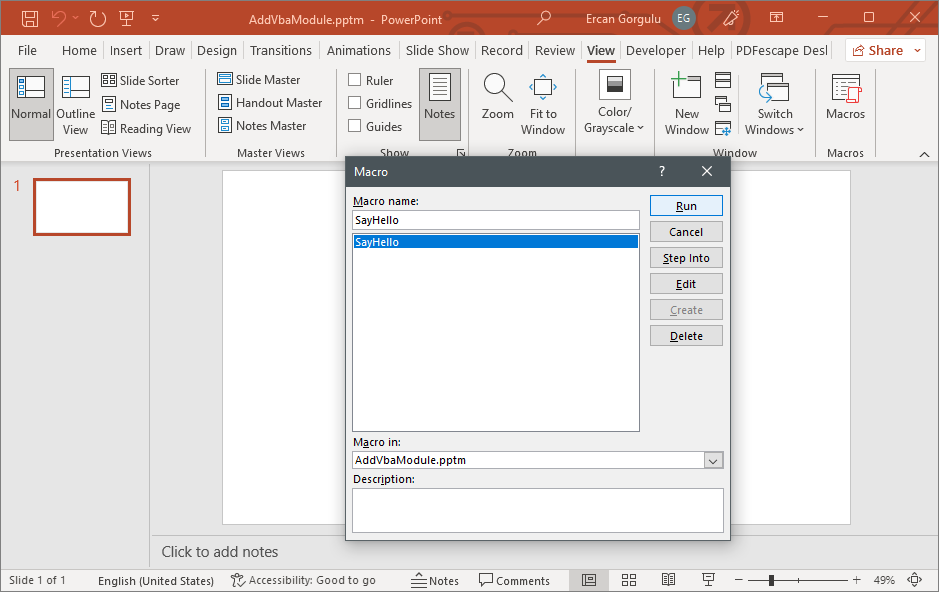
using GemBox.Presentation;
using GemBox.Presentation.Vba;
class Program
{
static void Main()
{
// If using Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
presentation.Slides.AddNew(SlideLayoutType.Custom);
// Create the module.
VbaModule vbaModule = presentation.VbaProject.Modules.Add("SampleModule");
vbaModule.Code =
@"Sub SayHello()
MsgBox ""Hello World!""
End Sub";
// Save the presentation as macro-enabled PowerPoint file.
presentation.Save("AddVbaModule.pptm");
}
}
Imports GemBox.Presentation
Imports GemBox.Presentation.Vba
Module Program
Sub Main()
' If using Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation As New PresentationDocument()
presentation.Slides.AddNew(SlideLayoutType.Custom)
' Create the module.
Dim vbaModule As VbaModule = presentation.VbaProject.Modules.Add("SampleModule")
vbaModule.Code =
"Sub SayHello()
MsgBox ""Hello World!""
End Sub"
' Save the presentation as macro-enabled PowerPoint file.
presentation.Save("AddVbaModule.pptm")
End Sub
End Module
The following example shows how you can update an PowerPoint VBA module in C# and VB.NET.
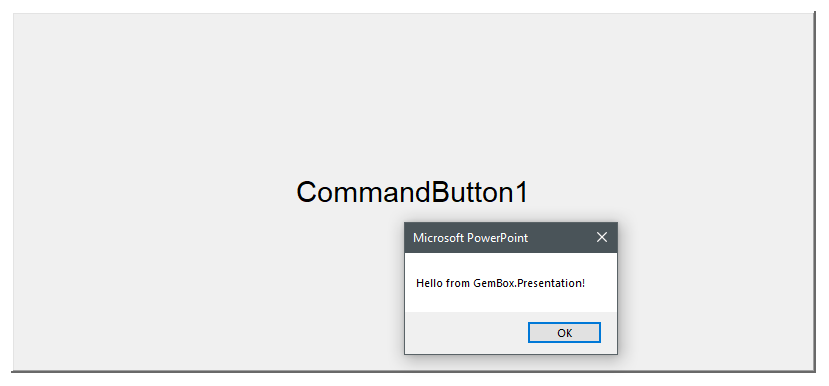
using GemBox.Presentation;
using GemBox.Presentation.Vba;
class Program
{
static void Main()
{
// If using Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = PresentationDocument.Load("%#SampleVba.pptm%");
// Get the module.
VbaModule vbaModule = presentation.VbaProject.Modules["Slide1"];
// Update text for the popup message.
vbaModule.Code = vbaModule.Code.Replace("Hello world!", "Hello from GemBox.Presentation!");
presentation.Save("UpdateVbaModule.pptm");
}
}
Imports GemBox.Presentation
Imports GemBox.Presentation.Vba
Module Program
Sub Main()
' If using Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = PresentationDocument.Load("%#SampleVba.pptm%")
' Get the module.
Dim vbaModule As VbaModule = presentation.VbaProject.Modules("Slide1")
' Update text for the popup message.
vbaModule.Code = vbaModule.Code.Replace("Hello world!", "Hello from GemBox.Presentation!")
presentation.Save("UpdateVbaModule.pptm")
End Sub
End Module