Rotate and flip images in Xamarin
The following example shows how to use GemBox.Imaging to rotate an image at 180 degrees and flip it vertically in a Xamarin.Forms mobile application.
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="ImagingXamarin.MainPage">
<StackLayout Padding="30"
Spacing="20">
<Label Text="GemBox.Imaging Example"
HorizontalOptions="Center"
FontSize="Large" />
<Picker x:Name="rotateFlipPicker" Title="Rotation/Flipping" ItemsSource="{Binding RotateFlipOptions}" ItemDisplayBinding="{Binding Text}" />
<Image x:Name="sourceImage" Source="" HeightRequest="160" WidthRequest="160" />
<Button x:Name="button"
Text="TRANSFORM IMAGE"
Clicked="Button_Clicked"/>
</StackLayout>
</ContentPage>
using GemBox.Imaging;
using ImagingXamarin.Models;
using System;
using System.IO;
using System.Net;
using Xamarin.Essentials;
using Xamarin.Forms;
public partial class MainPage : ContentPage
{
private byte[] imageData;
public MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
InitializeComponent();
BindingContext = new RotateFlipViewModel();
}
protected override void OnAppearing()
{
base.OnAppearing();
if (imageData == null)
using (var client = new WebClient())
this.imageData = client.DownloadData("https://www.gemboxsoftware.com/imaging/examples/101/resources/FragonardReader.jpg");
this.sourceImage.Source = ImageSource.FromStream(() => new MemoryStream(this.imageData));
}
private string RotateImage(Stream stream)
{
var documentPath = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
var selectedRotationFlipping = (RotateFlipOption)rotateFlipPicker.SelectedItem;
var destinationFilePath = Path.Combine(documentPath, $"{selectedRotationFlipping.Text}.jpg");
using (var image = GemBox.Imaging.Image.Load(stream))
{
image.RotateFlip(selectedRotationFlipping.Type);
image.Save(destinationFilePath);
}
return destinationFilePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
try
{
var filePath = RotateImage(new MemoryStream(this.imageData));
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
button.IsEnabled = true;
}
public class RotateFlipOption
{
public RotateFlipType Type { get; set; }
public string Text { get; set; }
}
public class RotateFlipViewModel
{
public ICollection<RotateFlipOption> RotateFlipOptions { get; set; }
public RotateFlipViewModel()
{
// Filling the collection for picker with possible RotateFlipTypes.
this.RotateFlipOptions = Enum.GetNames(typeof(RotateFlipType))
.Select(x => new RotateFlipOption
{
Text = x,
Type = (RotateFlipType)Enum.Parse(typeof(RotateFlipType), x)
}).ToList();
}
}
}
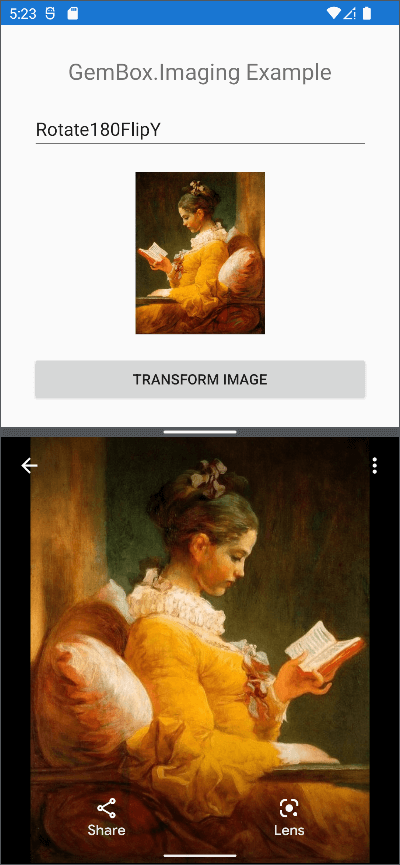