Merge and split image frames
With GemBox.Imaging, you can merge image files programmatically in C# and VB.NET, as shown in the following example.
using GemBox.Imaging;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Get all images from a folder.
var imagesEnumerable = Directory.EnumerateFiles("Frames").Select(Image.Load);
// Merge images into a single image.
using (var image = new Image(imagesEnumerable, true))
{
// Set infinite GIF loop count and add 1 second delay after the last frame.
image.Metadata.Gif.RepeatCount = 0;
image.Frames.Last().Metadata.Gif.FrameDelay = TimeSpan.FromSeconds(1);
// Save the merged image as a GIF file.
image.Save("Merged.gif");
}
}
}
Imports GemBox.Imaging
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Get all images from a folder.
Dim imagesEnumerable = Directory.EnumerateFiles("Frames").Select(Function(f) Image.Load(f))
' Merge images into a single image.
Using image = New Image(imagesEnumerable, True)
' Set infinite GIF loop count and add 1 second delay after the last frame.
image.Metadata.Gif.RepeatCount = 0
image.Frames.Last().Metadata.Gif.FrameDelay = TimeSpan.FromSeconds(1)
' Save the merged image as a GIF file.
image.Save("Merged.gif")
End Using
End Sub
End Module
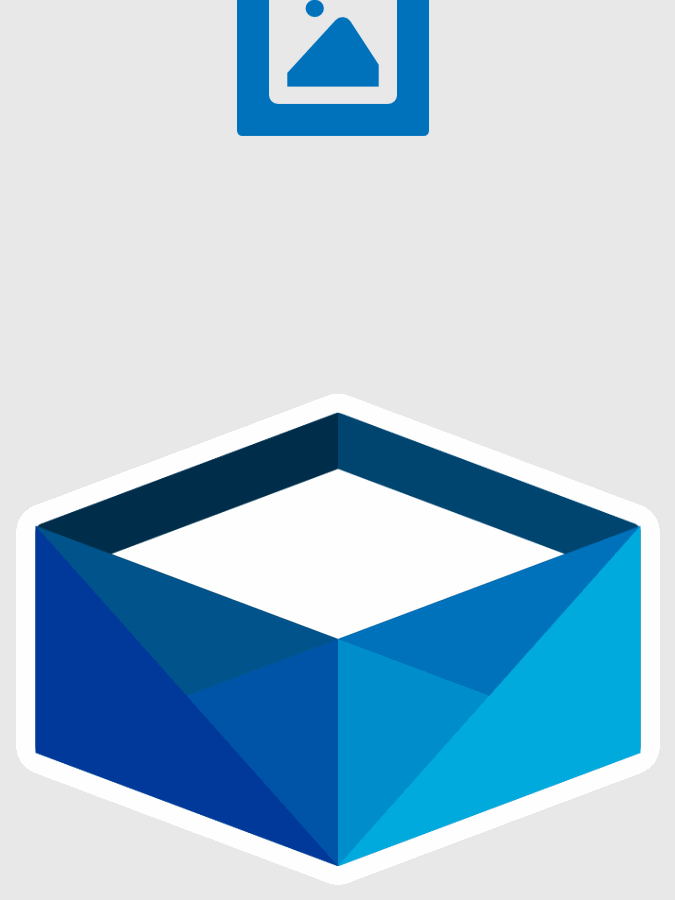
To merge images all you need to do is call the Image(images, disposeAfterLoad)
constructor with the collection of source images.
Split image
To split an image using GemBox.Imaging you need to load an image and loop through all frames exposed by the Image.Frames
property.
using GemBox.Imaging;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var imagePath = "%InputFileName%";
var frameIndex = %FrameIndex%;
// Load the input image and check if the requested frame exists.
using (var image = Image.Load(imagePath))
if (frameIndex < image.Frames.Count)
{
// Create an image from the frame at the specified index and save it to a new file.
using (var tempImage = new Image(image.Frames[frameIndex]))
tempImage.Save($"frame.{frameIndex + 1:D04}.%OutputFileType%");
}
}
}
Imports GemBox.Imaging
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim imagePath = "%InputFileName%"
Dim frameIndex = %FrameIndex%
' Load the input image and check if the requested frame exists.
Using image As Image = Image.Load(imagePath)
If frameIndex < image.Frames.Count Then
' Create an image from the frame at the specified index and save it to a new file.
Using tempImage = New Image(image.Frames(frameIndex))
tempImage.Save($"frame.{frameIndex + 1:D04}.png")
End Using
End If
End Using
End Sub
End Module
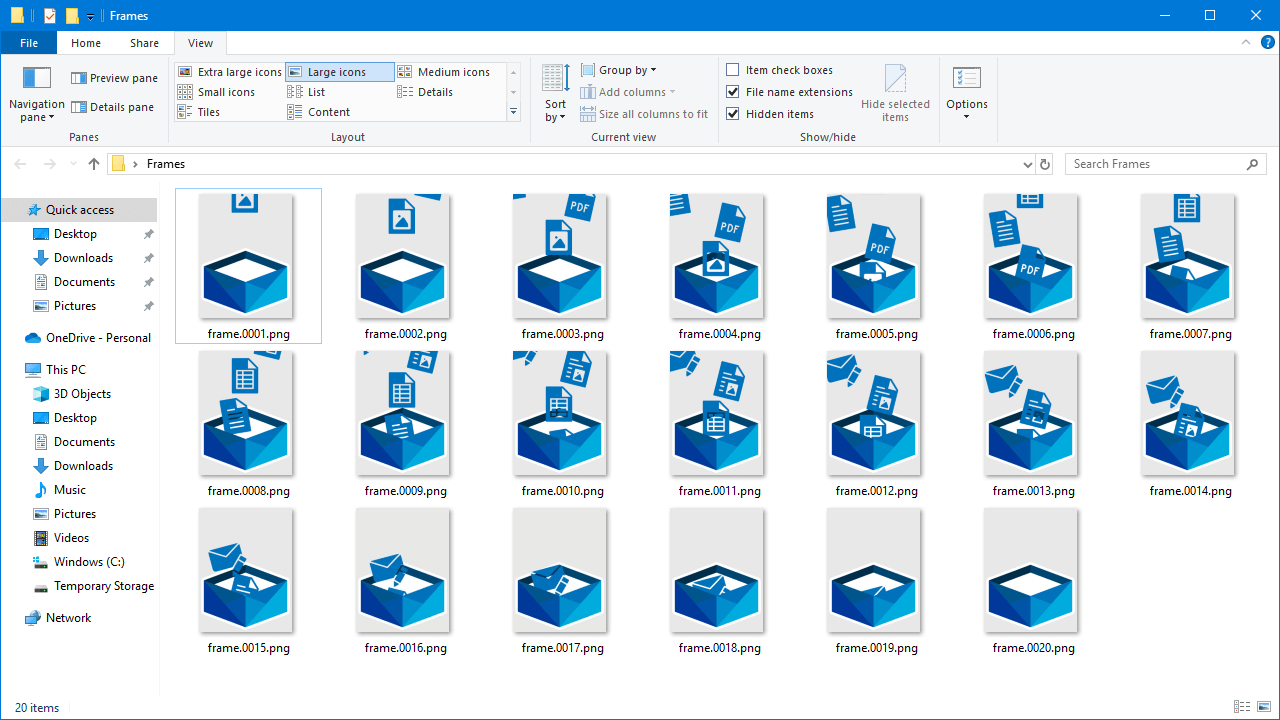