Convert ASPX to PDF in C# and VB.NET
When you need to convert ASPX web pages to PDF files within your application, the easiest and fastest way is to use a .NET library for PDF rendering.
With GemBox.Document you can convert most content types from ASPX to HTML and render them as PDF files. You can convert any texts, lists, tables, images, forms, and page layouts to look almost identical to the web page in the browser.
In this article, you will learn how to properly convert ASP.NET web pages to HTML and render them to PDF.
How to convert ASPX to PDF
The following example demonstrates how to convert an ASP.NET web page (a simple "About.aspx" page) to a PDF format and stream (download) the generated PDF file to the client's browser.
This is achieved by using a Page.Render method, in the code behind the desired page, to obtain ASPX content in HTML format.
The HTML formatted content is then loaded into a new document, and that document is written, in PDF format, directly to HttpResponse.OutputStream.
ASPX source web page
The following screenshot shows an ASP.NET web page that is converted to PDF. Notice that it contains a button control ("Convert ASPX page to PDF") which on click event downloads the PDF file created by converting the page's ASPX code.
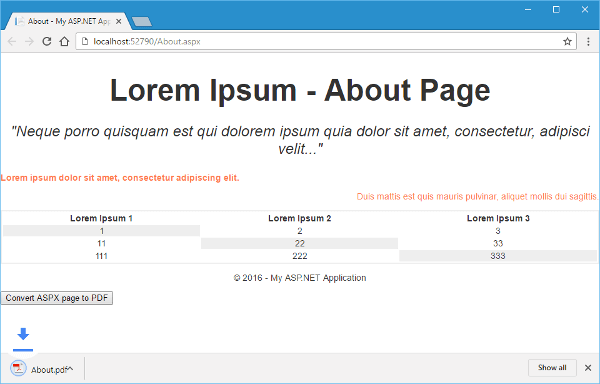
The following screenshot shows the resulting PDF file.
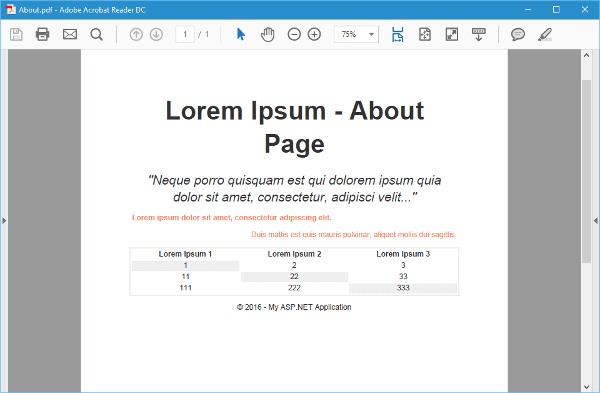
ASPX code
The following code is the content of the "About.aspx" file.
<%@ Page Title="About"
Language="C#"
MasterPageFile="~/Site.Master"
AutoEventWireup="true"
CodeBehind="About.aspx.cs"
Inherits="WebApplication.About"
EnableEventValidation="false" %>
<asp:Content ID="BodyContent" ContentPlaceHolderID="MainContent" runat="server">
<div style="font-family:Arial;font-size:14px;color:#333;text-align:center;">
<h1 style="font-size:48px;font-weight: bold;margin-bottom:20px;">
Lorem Ipsum - <%: Title %> Page
</h1>
<p style="font-size:24px;">
<em>"Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit..."</em>
</p>
<p style="color:coral;text-align:left;">
<strong>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</strong>
</p>
<p style="color:coral;text-align:right;">
Duis mattis est quis mauris pulvinar, aliquet mollis dui sagittis.
</p>
<table style="width:100%;border:2px solid #EEE;">
<tr><th>Lorem Ipsum 1</th><th>Lorem Ipsum 2</th><th>Lorem Ipsum 3</th></tr>
<tr><td style="background-color:#EEE;">1</td><td>2</td><td>3</td></tr>
<tr><td>11</td><td style="background-color:#EEE;">22</td><td>33</td></tr>
<tr><td>111</td><td>222</td><td style="background-color:#EEE;">333</td></tr>
</table>
<p>© <%: DateTime.Now.Year %> - My ASP.NET Application</p>
</div>
<asp:Button ID="Button1" runat="server" Text="Convert ASPX page to PDF" OnClick="Button1_Click" />
</asp:Content>
Notice that the page's EnableEventValidation
attribute is set to "false". This is required to avoid a runtime error when calling the Page.Render method.
C# code
In The following code is the content of the "About.aspx.cs" file, the page's code in C# language.
using System;
using System.IO;
using System.Web.UI;
using GemBox.Document;
public partial class About : Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Set license key to use GemBox.Document in a Free mode.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Continue to use the component in a Trial mode when free limit is reached.
ComponentInfo.FreeLimitReached += (sen, ev) => ev.FreeLimitReachedAction = FreeLimitReachedAction.ContinueAsTrial;
}
protected void Button1_Click(object sender, EventArgs e)
{
// Render ASPX page as HTML formatted string.
StringWriter sw = new StringWriter();
HtmlTextWriter htw = new HtmlTextWriter(sw);
this.Render(htw);
// Load HTML text to DocumentModel.
string html = sw.ToString();
DocumentModel document = new DocumentModel();
document.Content.LoadText(html, LoadOptions.HtmlDefault);
// Convert ASPX to PDF by exporting, downloading,
// DocumentModel in PDF format from ASP.NET application.
document.Save(this.Response, "About.pdf");
}
// Required to avoid the runtime error.
public override void VerifyRenderingInServerForm(Control control)
{
}
}
VB.NET code
The following code is the content of "About.aspx.vb" file, the page's code behind in VB.NET language.
Imports System
Imports System.IO
Imports System.Web.UI
Imports GemBox.Document
Partial Public Class About
Inherits Page
Protected Sub Page_Load(sender As Object, e As EventArgs)
' Set license key to use GemBox.Document in a Free mode.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Continue to use the component in a Trial mode when free limit is reached.
AddHandler ComponentInfo.FreeLimitReached, Sub(sen, ev) ev.FreeLimitReachedAction = FreeLimitReachedAction.ContinueAsTrial
End Sub
Protected Sub Button1_Click(sender As Object, e As EventArgs)
' Render ASPX page as HTML formatted string.
Dim sw As New StringWriter()
Dim htw As New HtmlTextWriter(sw)
Me.Render(htw)
' Load HTML text to DocumentModel.
Dim html As String = sw.ToString()
Dim document As New DocumentModel()
document.Content.LoadText(html, LoadOptions.HtmlDefault)
' Convert ASPX to PDF by exporting, downloading,
' DocumentModel in PDF format from ASP.NET application.
document.Save(Me.Response, "About.pdf")
End Sub
' Required to avoid the runtime error.
Public Overrides Sub VerifyRenderingInServerForm(control As Control)
End Sub
End Class
For a more detailed example, see Convert Word and HTML to PDF in C# and VB.NET from GemBox.Document Examples.