Character Formatting
Character formatting is used to enhance the appearance of the document's text. GemBox.Document provides this type of formatting through the CharacterFormat
class.
You have various formatting options at your disposal, like font name, font size, font color, bold, italic, underline, subscript, superscript, and many more. The formatting can be set directly on the text elements by using properties like Run.CharacterFormat
and Field.CharacterFormat
.
The following example shows all available character formatting options.
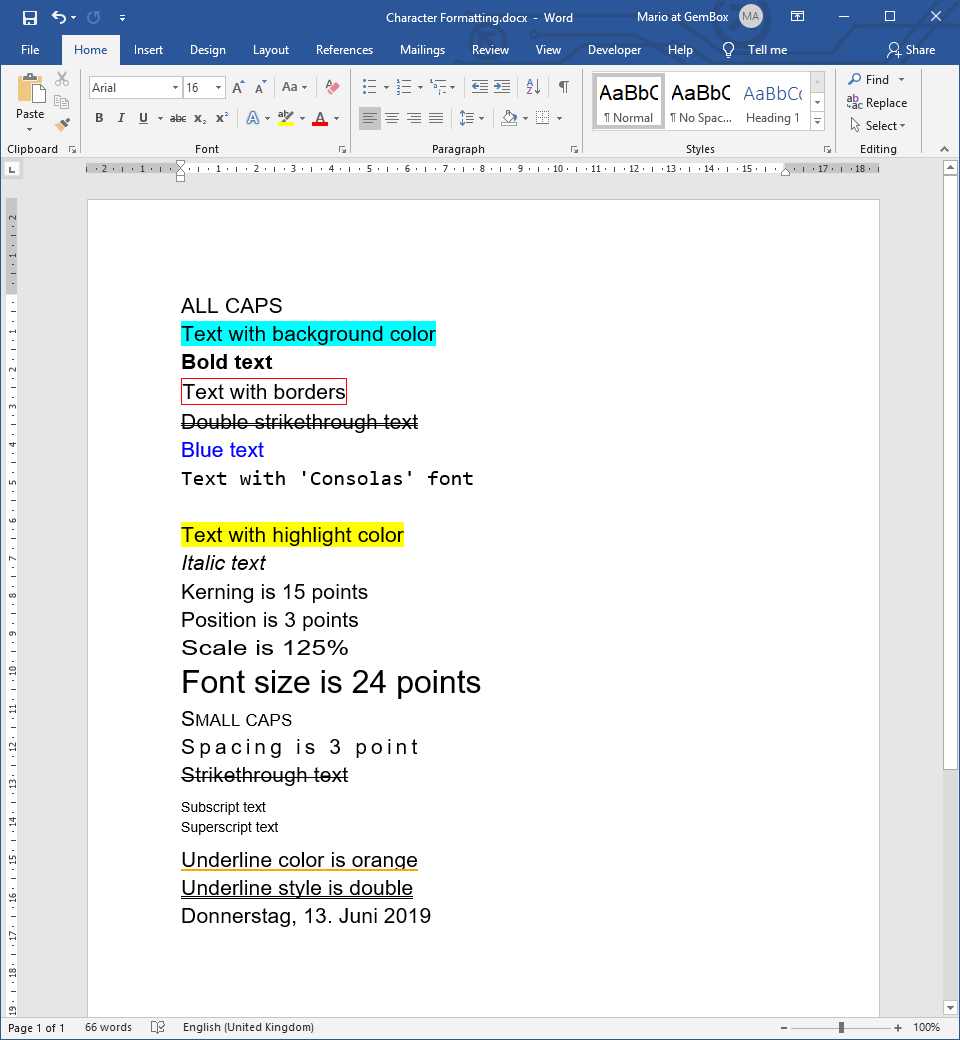
using System.Globalization;
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
DocumentModel document = new DocumentModel();
document.DefaultCharacterFormat.FontName = "Arial";
document.DefaultCharacterFormat.Size = 16;
SpecialCharacter lineBreakElement = new SpecialCharacter(document, SpecialCharacterType.LineBreak);
document.Sections.Add(
new Section(document,
new Paragraph(document,
new Run(document, "All caps") { CharacterFormat = { AllCaps = true } },
lineBreakElement,
new Run(document, "Text with background color") { CharacterFormat = { BackgroundColor = Color.Cyan } },
lineBreakElement.Clone(),
new Run(document, "Bold text") { CharacterFormat = { Bold = true } },
lineBreakElement.Clone(),
new Run(document, "Text with borders") { CharacterFormat = { Border = new SingleBorder(BorderStyle.Single, Color.Red, 1) } },
lineBreakElement.Clone(),
new Run(document, "Double strikethrough text") { CharacterFormat = { DoubleStrikethrough = true } },
lineBreakElement.Clone(),
new Run(document, "Blue text") { CharacterFormat = { FontColor = Color.Blue } },
lineBreakElement.Clone(),
new Run(document, "Text with 'Consolas' font") { CharacterFormat = { FontName = "Consolas" } },
lineBreakElement.Clone(),
new Run(document, "Hidden text") { CharacterFormat = { Hidden = true } },
lineBreakElement.Clone(),
new Run(document, "Text with highlight color") { CharacterFormat = { HighlightColor = Color.Yellow } },
lineBreakElement.Clone(),
new Run(document, "Italic text") { CharacterFormat = { Italic = true } },
lineBreakElement.Clone(),
new Run(document, "Kerning is 15 points") { CharacterFormat = { Kerning = 15 } },
lineBreakElement.Clone(),
new Run(document, "Position is 3 points") { CharacterFormat = { Position = 3 } },
lineBreakElement.Clone(),
new Run(document, "Scale is 125%") { CharacterFormat = { Scaling = 125 } },
lineBreakElement.Clone(),
new Run(document, "Font size is 24 points") { CharacterFormat = { Size = 24 } },
lineBreakElement.Clone(),
new Run(document, "Small caps") { CharacterFormat = { SmallCaps = true } },
lineBreakElement.Clone(),
new Run(document, "Spacing is 3 point") { CharacterFormat = { Spacing = 3 } },
lineBreakElement.Clone(),
new Run(document, "Strikethrough text") { CharacterFormat = { Strikethrough = true } },
lineBreakElement.Clone(),
new Run(document, "Subscript text") { CharacterFormat = { Subscript = true } },
lineBreakElement.Clone(),
new Run(document, "Superscript text") { CharacterFormat = { Superscript = true } },
lineBreakElement.Clone(),
new Run(document, "Underline color is orange") { CharacterFormat = { UnderlineColor = Color.Orange, UnderlineStyle = UnderlineType.Single } },
lineBreakElement.Clone(),
new Run(document, "Underline style is double") { CharacterFormat = { UnderlineStyle = UnderlineType.Double } },
lineBreakElement.Clone(),
new Field(document, FieldType.Date, @"\@ ""dddd, d. MMMM yyyy""") { CharacterFormat = { Language = CultureInfo.GetCultureInfo("de-DE") } })));
document.Save("Character Formatting.%OutputFileType%");
}
}
Imports System.Globalization
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
document.DefaultCharacterFormat.FontName = "Arial"
document.DefaultCharacterFormat.Size = 16
Dim lineBreakElement As New SpecialCharacter(document, SpecialCharacterType.LineBreak)
document.Sections.Add(
New Section(document,
New Paragraph(document,
New Run(document, "All caps") With {.CharacterFormat = New CharacterFormat With {.AllCaps = True}},
lineBreakElement,
New Run(document, "Text with background color") With {.CharacterFormat = New CharacterFormat With {.BackgroundColor = Color.Cyan}},
lineBreakElement.Clone(),
New Run(document, "Bold text") With {.CharacterFormat = New CharacterFormat With {.Bold = True}},
lineBreakElement.Clone(),
New Run(document, "Text with borders") With {.CharacterFormat = New CharacterFormat With {.Border = New SingleBorder(BorderStyle.Single, Color.Red, 1)}},
lineBreakElement.Clone(),
New Run(document, "Double strikethrough text") With {.CharacterFormat = New CharacterFormat With {.DoubleStrikethrough = True}},
lineBreakElement.Clone(),
New Run(document, "Blue text") With {.CharacterFormat = New CharacterFormat With {.FontColor = Color.Blue}},
lineBreakElement.Clone(),
New Run(document, "Text with 'Consolas' font") With {.CharacterFormat = New CharacterFormat With {.FontName = "Consolas"}},
lineBreakElement.Clone(),
New Run(document, "Hidden text") With {.CharacterFormat = New CharacterFormat With {.Hidden = True}},
lineBreakElement.Clone(),
New Run(document, "Text with highlight color") With {.CharacterFormat = New CharacterFormat With {.HighlightColor = Color.Yellow}},
lineBreakElement.Clone(),
New Run(document, "Italic text") With {.CharacterFormat = New CharacterFormat With {.Italic = True}},
lineBreakElement.Clone(),
New Run(document, "Kerning is 15 points") With {.CharacterFormat = New CharacterFormat With {.Kerning = 15}},
lineBreakElement.Clone(),
New Run(document, "Position is 3 points") With {.CharacterFormat = New CharacterFormat With {.Position = 3}},
lineBreakElement.Clone(),
New Run(document, "Scale is 125%") With {.CharacterFormat = New CharacterFormat With {.Scaling = 125}},
lineBreakElement.Clone(),
New Run(document, "Font size is 24 points") With {.CharacterFormat = New CharacterFormat With {.Size = 24}},
lineBreakElement.Clone(),
New Run(document, "Small caps") With {.CharacterFormat = New CharacterFormat With {.SmallCaps = True}},
lineBreakElement.Clone(),
New Run(document, "Spacing is 3 point") With {.CharacterFormat = New CharacterFormat With {.Spacing = 3}},
lineBreakElement.Clone(),
New Run(document, "Strikethrough text") With {.CharacterFormat = New CharacterFormat With {.Strikethrough = True}},
lineBreakElement.Clone(),
New Run(document, "Subscript text") With {.CharacterFormat = New CharacterFormat With {.Subscript = True}},
lineBreakElement.Clone(),
New Run(document, "Superscript text") With {.CharacterFormat = New CharacterFormat With {.Superscript = True}},
lineBreakElement.Clone(),
New Run(document, "Underline color is orange") With {.CharacterFormat = New CharacterFormat With {.UnderlineColor = Color.Orange, .UnderlineStyle = UnderlineType.Single}},
lineBreakElement.Clone(),
New Run(document, "Underline style is double") With {.CharacterFormat = New CharacterFormat With {.UnderlineStyle = UnderlineType.Double}},
lineBreakElement.Clone(),
New Field(document, FieldType.Date, "\@ ""dddd, d. MMMM yyyy""") With {.CharacterFormat = New CharacterFormat With {.Language = CultureInfo.GetCultureInfo("de-DE")}})))
document.Save("Character Formatting.%OutputFileType%")
End Sub
End Module
Direct formatting has the highest priority in format resolution. If it's not present, then the formatting can be retrieved from a style that's applied to an element (CharacterStyle.CharacterFormat
).
If there is no style, then the formatting can be retrieved from the document's default formatting (DocumentModel.DefaultCharacterFormat
).
You can read more about this in the Style Resolution example or on the Formatting properties resolution help page.