PDF Encryption in C# and VB.NET
PDF encryption enables you to securely protect the content of your PDF document from unwanted viewers and against unwanted actions like printing, selecting text, and modifying annotations.
GemBox.Document will encrypt the output PDF file if you specify any permission different than PdfPermissions.All
or if either user password (DocumentOpenPassword
) or owner password (PermissionsPassword
) is not null
and empty
.
You can read more about this on the PdfSaveOptions.Permissions
remarks.
The following example shows how you can create an encrypted PDF file in C# and VB.NET with restricted permissions, which requires a password to be decrypted, viewed, and optionally modified.
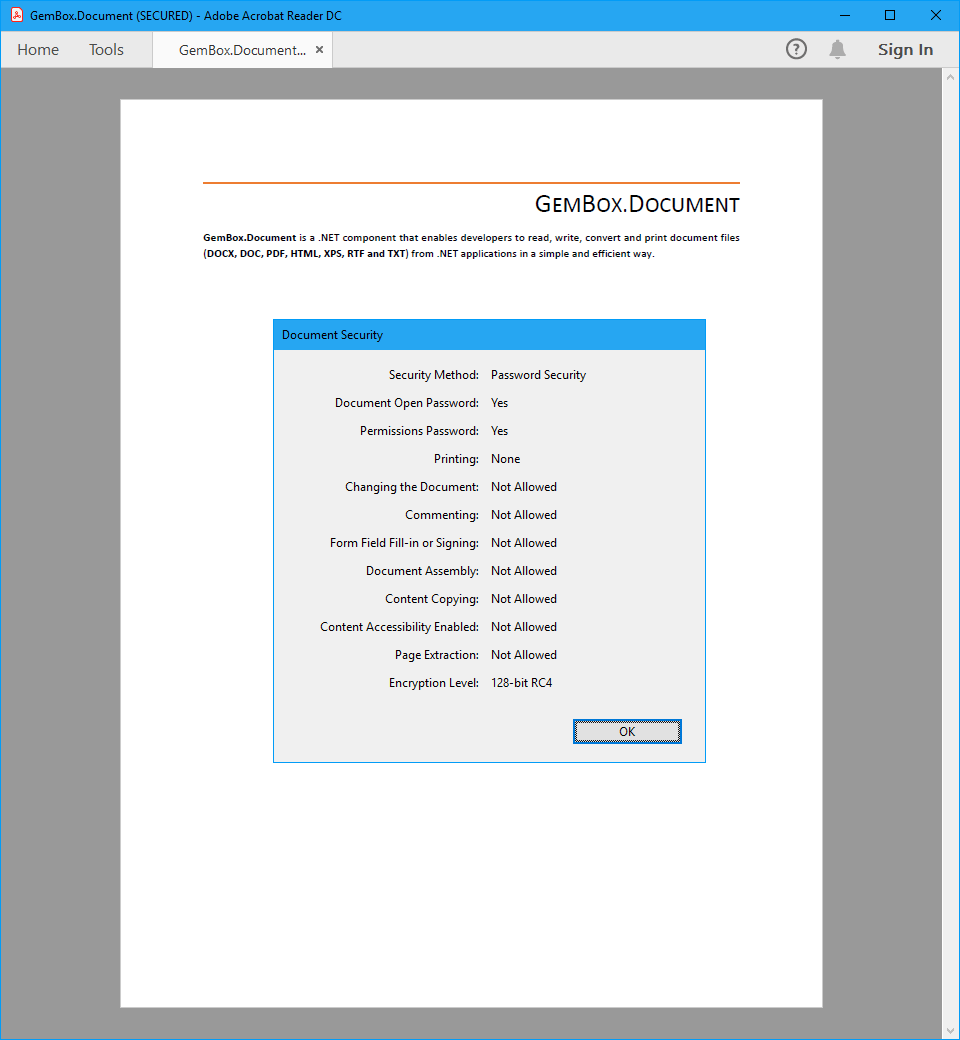
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
string userPassword = "%UserPassword%";
string ownerPassword = "%OwnerPassword%";
PdfPermissions permissions = %PdfPermissions%;
var document = DocumentModel.Load("%#Reading.docx%");
var options = new PdfSaveOptions()
{
DocumentOpenPassword = userPassword,
PermissionsPassword = ownerPassword,
Permissions = permissions
};
document.Save("PDF Encryption.pdf", options);
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim userPassword As String = "%UserPassword%"
Dim ownerPassword As String = "%OwnerPassword%"
Dim permissions As PdfPermissions = %PdfPermissions%
Dim document = DocumentModel.Load("%#Reading.docx%")
Dim options As New PdfSaveOptions() With
{
.DocumentOpenPassword = userPassword,
.PermissionsPassword = ownerPassword,
.Permissions = permissions
}
document.Save("PDF Encryption.pdf", options)
End Sub
End Module
Besides writing encrypted PDF, GemBox.Document also supports reading protected PDF files by decrypting them with the provided PdfLoadOptions.Password
value.
You can check Extract Text from PDF example to read more about GemBox.Document's PDF reading capabilities.
Nevertheless, in most cases when working with existing PDF files you'll find that a more appropriate approach is to use GemBox.Pdf to encrypt or decrypt the PDF.