Find and Replace text in a Word file with C# and VB.NET
GemBox.Document offers several ways in which you can manipulate a Word document's content to import new or replace existing data using C# or VB.NET code. For instance, you can use Mail Merge, Update Form, Content Controls Mapping, Modify Word Bookmarks, or the following find and replace approach.
You can easily find all the parts of a Word document that contain the specified text or match the specified regular expression and replace them with desired text by using one of the ContentRange.Replace
methods.
You can also search for all occurrences of a specified String
or Regex
using one of the ContentRange.Find
methods and process the resulting ContentRange
objects as needed. This approach is useful when you need a more complex replacement, like replacing your placeholders with hyperlinks, tables, pictures, or some other content.
The following example shows how you can search for all occurrences of some placeholder text in a document and highlight or replace them with any desired text and optional formatting.
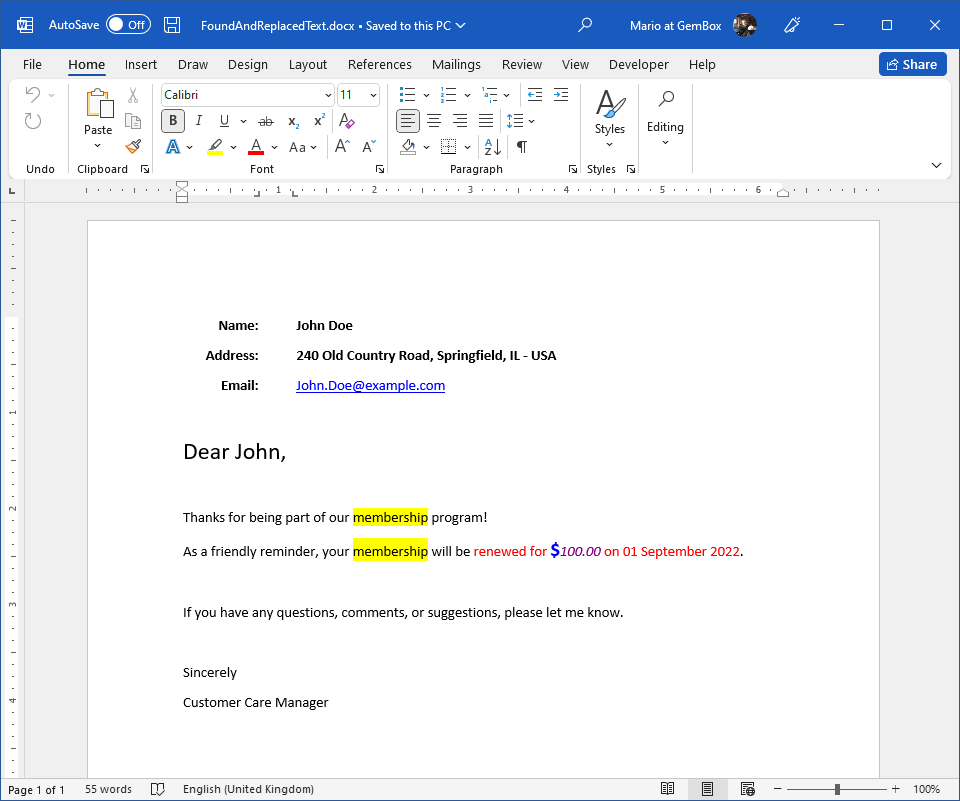
using GemBox.Document;
using System;
using System.Linq;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
// The easiest way how you can find and replace text is with "Replace" method.
document.Content.Replace("%FirstName%", "John");
document.Content.Replace("%LastName%", "Doe");
// Another way would be to use Regex.
document.Content.Replace(new Regex("%DATE%", RegexOptions.IgnoreCase),
DateTime.Today.ToLongDateString());
document.Content.Replace(new Regex("%.*?%"), range =>
{
string value = null;
switch (range.ToString())
{
case "%Address%": value = "240 Old Country Road"; break;
case "%City%": value = "Springfield"; break;
case "%State%": value = "IL"; break;
case "%Country%": value = "USA"; break;
};
if (string.IsNullOrEmpty(value))
return range;
var format = ((Run)range.Start.Parent).CharacterFormat;
var run = new Run(document, value) { CharacterFormat = format.Clone() };
return run.Content;
});
// You can also search for placeholder text with the "Find" method and then achieve a
// more complex replacement, like the following which has a replace text with different formatting.
// Notice that the "Reverse" extension method is used here to avoid a possible invalid state because
// the replacements are done while iterating through the document's content.
foreach (ContentRange searchedContent in document.Content.Find("%Price%").Reverse())
{
ContentRange replacedContent = searchedContent.LoadText("$",
new CharacterFormat() { Size = 14, FontColor = Color.Blue, Bold = true });
replacedContent.End.LoadText("100.00",
new CharacterFormat() { Size = 11, FontColor = Color.Purple, Italic = true });
}
// Another more complex replacement in which searched text is replaced with a hyperlink.
foreach (ContentRange searchedContent in document.Content.Find("%Email%").Reverse())
{
Hyperlink emailLink = new Hyperlink(document, "mailto:john.doe@example.com", "John.Doe@example.com");
searchedContent.Set(emailLink.Content);
}
// You can also find and highlight text by specifying "HighlightColor" of replacement text.
foreach (ContentRange searchedContent in document.Content.Find("membership").Reverse())
{
var highlightedText = new Run(document, "membership");
highlightedText.CharacterFormat = ((Run)searchedContent.Start.Parent).CharacterFormat.Clone();
highlightedText.CharacterFormat.HighlightColor = Color.Yellow;
searchedContent.Set(highlightedText.Content);
}
document.Save("FoundAndReplacedText.%OutputFileType%");
}
}
Imports GemBox.Document
Imports System
Imports System.Linq
Imports System.Text.RegularExpressions
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%InputFileName%")
' The easiest way how you can find and replace text is with "Replace" method.
document.Content.Replace("%FirstName%", "John")
document.Content.Replace("%LastName%", "Doe")
' Another way would be to use Regex.
document.Content.Replace(New Regex("%DATE%", RegexOptions.IgnoreCase),
DateTime.Today.ToLongDateString())
document.Content.Replace(New Regex("%.*?%"),
Function(range)
Dim value As String = Nothing
Select Case range.ToString()
Case "%Address%"
value = "240 Old Country Road"
Case "%City%"
value = "Springfield"
Case "%State%"
value = "IL"
Case "%Country%"
value = "USA"
End Select
If String.IsNullOrEmpty(value) Then Return range
Dim format = (CType(range.Start.Parent, Run)).CharacterFormat
Dim run As New Run(document, value) With {.CharacterFormat = format.Clone()}
Return run.Content
End Function)
' You can also search for placeholder text with the "Find" method and then achieve a
' more complex replacement, like the following which has a replace text with different formatting.
' Notice that the "Reverse" extension method is used here to avoid a possible invalid state because
' the replacements are done while iterating through the document's content.
For Each searchedContent As ContentRange In document.Content.Find("%Price%").Reverse()
Dim replacedContent As ContentRange = searchedContent.LoadText("$",
New CharacterFormat() With {.Size = 14, .FontColor = Color.Blue, .Bold = True})
replacedContent.End.LoadText("100.00",
New CharacterFormat() With {.Size = 11, .FontColor = Color.Purple, .Italic = True})
Next
' Another more complex replacement in which searched text is replaced with a hyperlink.
For Each searchedContent As ContentRange In document.Content.Find("%Email%").Reverse()
Dim emailLink As New Hyperlink(document, "mailto:john.doe@example.com", "John.Doe@example.com")
searchedContent.Set(emailLink.Content)
Next
' You can also find and highlight text by specifying "HighlightColor" of replacement text.
For Each searchedContent As ContentRange In document.Content.Find("membership").Reverse()
Dim highlightedText As New Run(document, "membership")
highlightedText.CharacterFormat = (CType(searchedContent.Start.Parent, Run)).CharacterFormat.Clone()
highlightedText.CharacterFormat.HighlightColor = Color.Yellow
searchedContent.Set(highlightedText.Content)
Next
document.Save("FoundAndReplacedText.%OutputFileType%")
End Sub
End Module
Instead of just replacing the document's text with another plain text, the following example shows how you can replace placeholder text in a Word document with Picture
and Table
elements.
Also, the following example shows how you can specify your replacement text in HTML format.
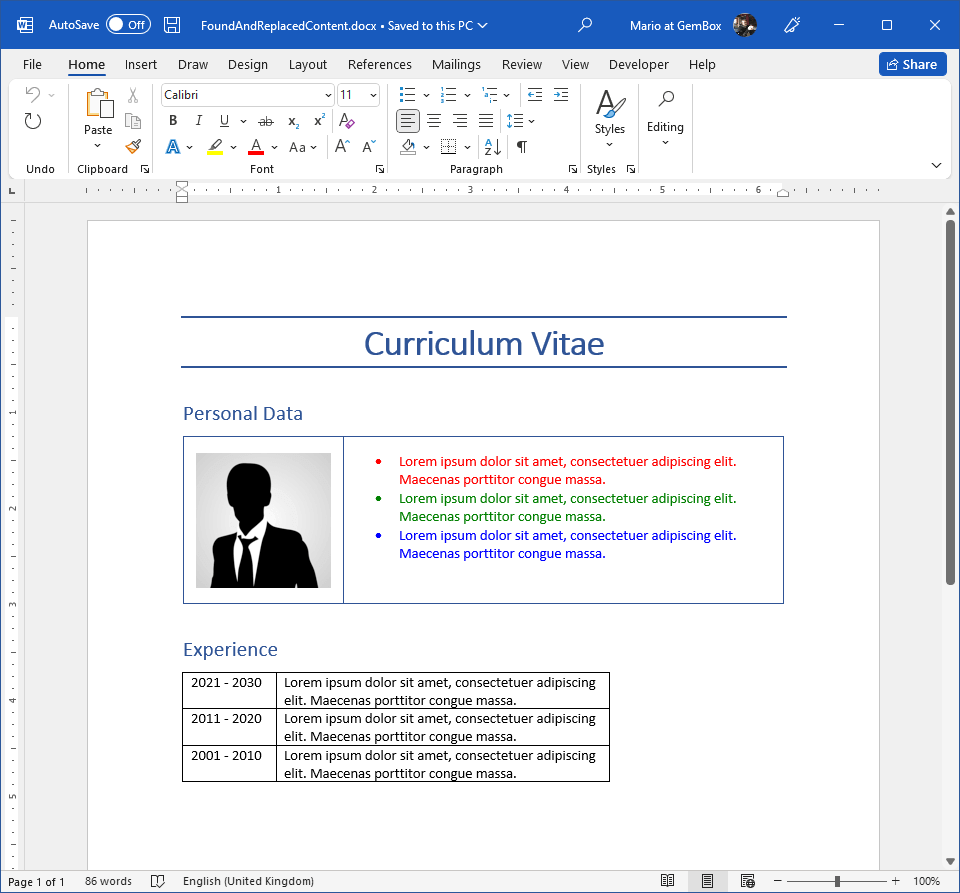
using GemBox.Document;
using GemBox.Document.Tables;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#FindAndReplaceContent.docx%");
var dummyText = "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Maecenas porttitor congue massa.";
// Find an image placeholder.
var picturePlaceholder = document.Content.Find("%Portrait%").First();
var picture = new Picture(document, "%#avatar.png%");
// Replace the placeholder text with image.
picturePlaceholder.Set(picture.Content);
// Find an HTML placeholder.
var htmlPlaceholder = document.Content.Find("%AboutMe%").First();
var html =
$@"<ul style='font:11pt Calibri;'>
<li style='color:red;'>{dummyText}</li>
<li style='color:green;'>{dummyText}</li>
<li style='color:blue;'>{dummyText}</li>
</ul>";
// Replace the placeholder text with HTML formatted text.
htmlPlaceholder.LoadText(html, new HtmlLoadOptions());
// Find a table placeholder.
var tablePlaceholder = document.Content.Find("%JobHistory%").First();
var table = new Table(document,
new TableRow(document,
new TableCell(document, new Paragraph(document, "2021 - 2030")),
new TableCell(document, new Paragraph(document, dummyText))),
new TableRow(document,
new TableCell(document, new Paragraph(document, "2011 - 2020")),
new TableCell(document, new Paragraph(document, dummyText))),
new TableRow(document,
new TableCell(document, new Paragraph(document, "2001 - 2010")),
new TableCell(document, new Paragraph(document, dummyText))));
table.Columns.Add(new TableColumn(70));
table.Columns.Add(new TableColumn(250));
table.TableFormat.AutomaticallyResizeToFitContents = false;
// Delete the placeholder text and insert the table before it.
tablePlaceholder = tablePlaceholder.LoadText(string.Empty);
tablePlaceholder.Start.InsertRange(table.Content);
document.Save("FoundAndReplacedContent.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Tables
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#FindAndReplaceContent.docx%")
Dim dummyText = "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Maecenas porttitor congue massa."
' Find an image placeholder.
Dim picturePlaceholder = document.Content.Find("%Portrait%").First()
Dim picture As New Picture(document, "%#avatar.png%")
' Replace the placeholder text with image.
picturePlaceholder.Set(picture.Content)
' Find an HTML placeholder.
Dim htmlPlaceholder = document.Content.Find("%AboutMe%").First()
Dim html =
$"<ul style='font:11pt Calibri;'>
<li style='color:red;'>{dummyText}</li>
<li style='color:green;'>{dummyText}</li>
<li style='color:blue;'>{dummyText}</li>
</ul>"
' Replace the placeholder text with HTML formatted text.
htmlPlaceholder.LoadText(html, New HtmlLoadOptions())
' Find a table placeholder.
Dim tablePlaceholder = document.Content.Find("%JobHistory%").First()
Dim table As New Table(document,
New TableRow(document,
New TableCell(document, New Paragraph(document, "2021 - 2030")),
New TableCell(document, New Paragraph(document, dummyText))),
New TableRow(document,
New TableCell(document, New Paragraph(document, "2011 - 2020")),
New TableCell(document, New Paragraph(document, dummyText))),
New TableRow(document,
New TableCell(document, New Paragraph(document, "2001 - 2010")),
New TableCell(document, New Paragraph(document, dummyText))))
table.Columns.Add(New TableColumn(70))
table.Columns.Add(New TableColumn(250))
table.TableFormat.AutomaticallyResizeToFitContents = False
' Delete the placeholder text and insert the table before it.
tablePlaceholder = tablePlaceholder.LoadText(String.Empty)
tablePlaceholder.Start.InsertRange(table.Content)
document.Save("FoundAndReplacedContent.%OutputFileType%")
End Sub
End Module